Hello friends, today we will discuss Send SMS Via Twilio In Apex Rest API Salesforce. We will send this SMS by using Twilio API. For this, we need to create Twilio account. Get the ACCOUNT SID and AUTH TOKEN for authentication.
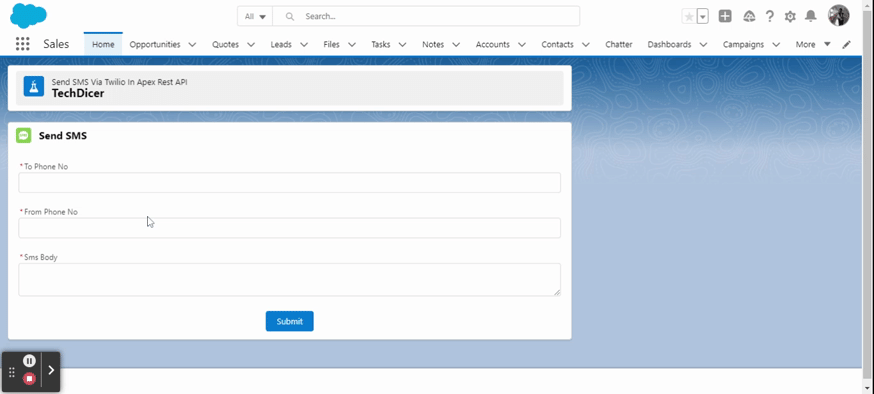
Highlights Points :
- Send SMS to given mobile no.
- Use Rest Api.
- Use Basic Auth for authenication.
- Twilio Salesforce Apex
- send SMS using Twilio in Salesforce
check this: Get Weather Info in Apex Rest API
Step 1: First we create Twilio Account and get the ACCOUNT SID and AUTH TOKEN for authentication. Click Here for creating an account. Twilio Web Link.
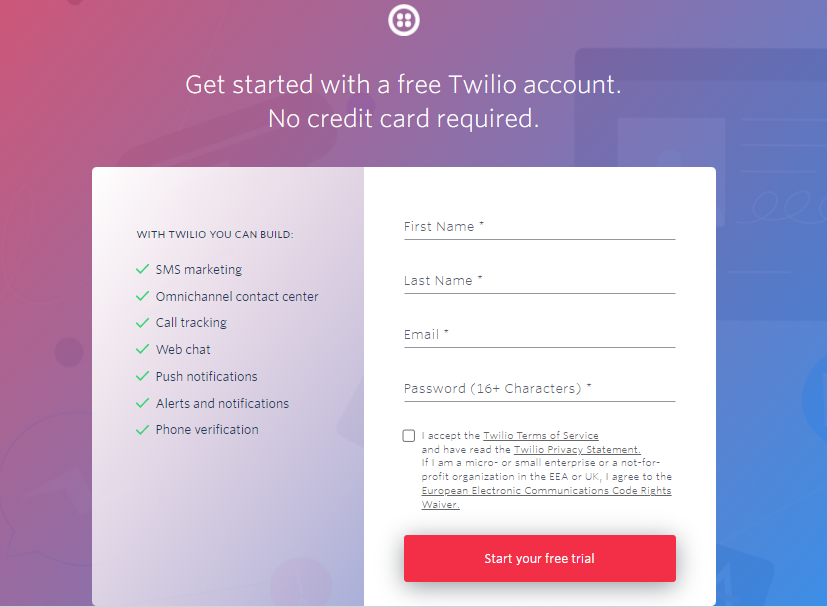
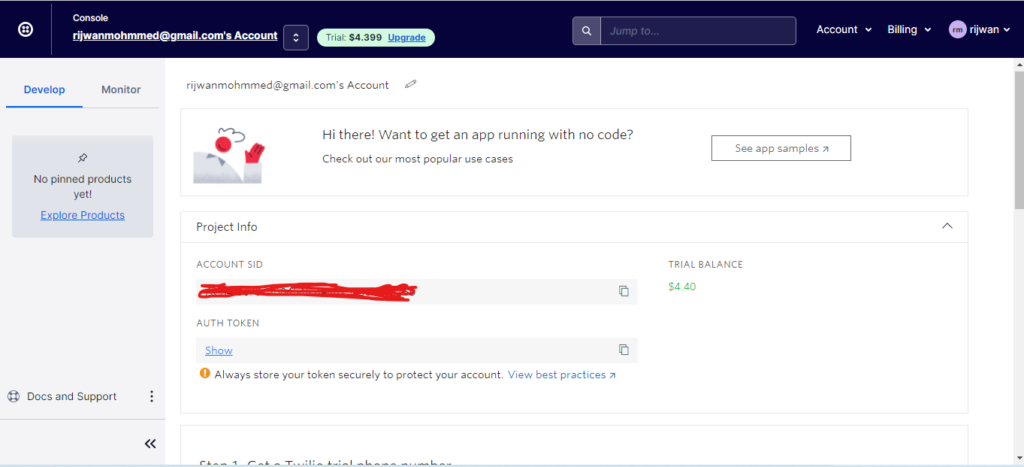
Step 2: in this step, we will do Remote Site Settings in salesforce org. Setup > Remote Site Settings > Click New
Create a Remote Site Settings in Salesforce for the below URL => https://api.twilio.com
Step 3: In this step, we will create an apex class and write the logic for sending SMS via Callout.
SendSMSCtrl.cls :
public class SendSMSCtrl {
@AuraEnabled
public static String SendSMS(String phoneNo, String smsBody, String fromPhNumber){
String accountSid = 'AC84f5c78c3b0454fc4e4XXXXXXXXX'; // you can put ACCOUNT SID from twilio account
String token = 'd5bf92de8ef4aabe4e1fXXXXXXXXXX'; // you can put AUTH TOKEN from twilio account
String endPoint = 'https://api.twilio.com/2010-04-01/Accounts/'+accountSid+'/SMS/Messages.json';
Blob creds = Blob.valueOf(accountSid+':' +token);
HttpRequest req = new HttpRequest();
Http http = new Http();
HTTPResponse res = new HTTPResponse();
req.setEndpoint(endPoint);
req.setMethod('POST');
String VERSION = '3.2.0';
req.setHeader('X-Twilio-Client', 'salesforce-' + VERSION);
req.setHeader('User-Agent', 'twilio-salesforce/' + VERSION);
req.setHeader('Accept', 'application/json');
req.setHeader('Accept-Charset', 'utf-8');
req.setHeader('Authorization', 'Basic '+EncodingUtil.base64Encode(creds));
req.setBody('To=' + EncodingUtil.urlEncode(phoneNo, 'UTF-8') + '&From=' + EncodingUtil.urlEncode(fromPhNumber,'UTF-8') + '&Body=' + smsBody);
res = http.send(req);
System.debug(res.getBody());
if(res.getStatusCode() == 201){
return 'SMS Sent Successfully';
} else{
errorWrapper er = (errorWrapper)json.deserialize(res.getBody(), errorWrapper.class);
throw newMessageException('Error : ' + er.message);
}
}
public class errorWrapper{
String code;
String message;
String moreInfo;
String status;
}
private static AuraHandledException newMessageException(String message) {
AuraHandledException e = new AuraHandledException(message);
e.setMessage(message);
return e;
}
}
Step 4: In this step, we create LWC Component for the UI part so users can interact and send SMS by putting phone no in input.
SendSMSLWC.Html :
<template>
<!-- loader -->
<div if:true={showSpinner}>
<lightning-spinner
alternative-text="Loading..." variant="brand">
</lightning-spinner>
</div>
<!------------->
<div class="slds-tabs_card">
<div class="slds-page-header">
<div class="slds-page-header__row">
<div class="slds-page-header__col-title">
<div class="slds-media">
<div class="slds-media__figure">
<span class="slds-icon_container slds-icon-standard-opportunity">
<lightning-icon icon-name="standard:recipe" alternative-text="recipe" title="recipe"></lightning-icon>
</span>
</div>
<div class="slds-media__body">
<div class="slds-page-header__name">
<div class="slds-page-header__name-title">
<h1>
<span>Send SMS Via Twilio In Apex Rest API</span>
<span class="slds-page-header__title slds-truncate" title="Recently Viewed">TechDicer</span>
</h1>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div> <br/>
<lightning-card title="Send SMS" icon-name="standard:sms">
<div class="slds-var-p-around_small">
<lightning-layout multiple-rows>
<lightning-layout-item padding="around-small" size="12" medium-device-size="12" large-device-size="12">
<lightning-input name="toPhoneNo" class="fieldvalidate" type="tel"
label="To Phone No" required>
</lightning-input><br/>
<lightning-input name="fromPhNumber" class="fieldvalidate" type="tel"
label="From Phone No" required>
</lightning-input><br/>
<lightning-textarea name="smsBody" label="Sms Body" required class="fieldvalidate">
</lightning-textarea>
</lightning-layout-item>
<lightning-layout-item size="12" class="slds-var-p-top_small">
<lightning-button class="slds-align_absolute-center" variant="brand" label="Submit"
onclick={sendSMS}></lightning-button>
</lightning-layout-item>
</lightning-layout>
</div>
</lightning-card><br/>
</template>
SendSMSLWC.JS :
import { LightningElement } from 'lwc';
import SendSMSCtrl from "@salesforce/apex/SendSMSCtrl.SendSMS";
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
export default class SendSMSLWC extends LightningElement {
smsBody;
toPhoneNo;
sendSMS(){
let inputFields = this.template.querySelectorAll('.fieldvalidate');
inputFields.forEach(inputField => {
if(inputField.name == "toPhoneNo"){
this.toPhoneNo = inputField.value;
} else if(inputField.name == "smsBody"){
this.smsBody = inputField.value;
} else if(inputField.name == "fromPhNumber"){
this.fromPhNumber = inputField.value;
}
});
this.handleSpinner();
//send data to server side to check wetaher
SendSMSCtrl({phoneNo : this.toPhoneNo, smsBody : this.smsBody, fromPhNumber : this.fromPhNumber})
.then(result => {
//do something
console.log(result);
const evt = new ShowToastEvent({
title: "Yikes!",
message: result,
variant: "success",
});
this.dispatchEvent(evt);
this.handleSpinner();
})
.catch((error) => {
//Let's send the user a toast with our custom error message
const evt = new ShowToastEvent({
title: "Yikes!",
message: error.body.message,
variant: "error",
});
this.dispatchEvent(evt);
this.handleSpinner();
})
}
handleSpinner(){
this.showSpinner = !this.showSpinner;
}
}
Step 5: for the testing, we need to setup “To Phone No” and From No. you can go account and set these by below links
Output :
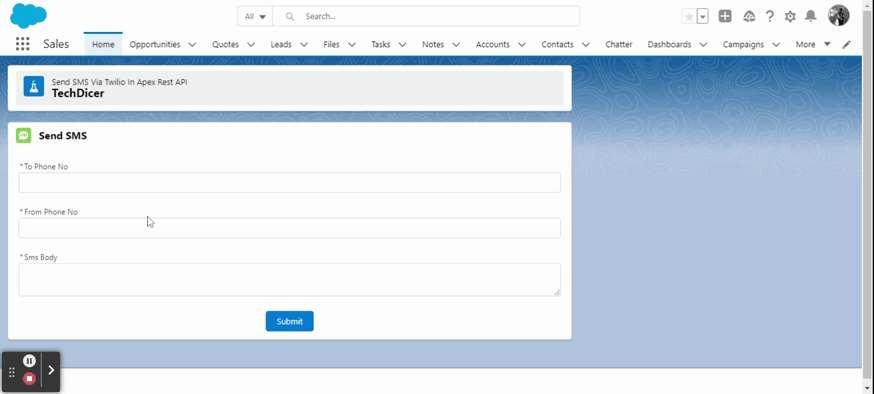
2 comments
Are there any steps left out above? I am not seeing my LWC components to add to my home page when I click “edit” on the home page.
Hi JASON, Have you added lightning home page in XML file