Hello friends, Today we are going to discuss Lightning Component Datatable with Sorting and pagination. Datatable is a table where we show the data in tabular form. When there are large data then we will show these data in chunks which is called pagination. We can set the page size to show it will break in chunks according to the size.
Also if we want the data in ascending or descending by a particular column of the table, we do sort the data so the data in Datatable is shown in a good way.
Check this: Pagination In Salesforce Lightning Web Components
Highlights Points :
- Datatble have pagination in which we will put NEXT, Previous buttons.
- It has sort the data by coumn wise.
- Retrive the data from apex class in Lightning Component.
- We can defiend column data type like number, url etc.
- Client Side Pagination
- Client Side Sorting
Let’s understand with an example.
Step 1: In this step, we create an apex class so we can call the methods from Lightning Component from apex class and fetch the data in. This method will be AuraEnabled annotation. We query the account data and return the accounts list.
LightningComponentSortingPagnation.cls :
public class LightningComponentSortingPagnation {
@AuraEnabled
public static List<Account> fetchAccountData(){
return [SELECT Id, Name, Type, Website, CreatedDate, Phone FROM Account LIMIT 1000];
}
}
Step 2: In this step, we create a Lightning component. First, we write code for Datatable and defined attributes to retain Datatable data. We defined a columns attribute so we can show the table accordingly. All columns are sortable=”true”. For pagination, we defined attributes like pagesize, totalrecords, currentPage, etc. For sorting the data we will call a method in the component controller by clicking on the column header.
LightningComponentSorting.cmp :
<aura:component implements="flexipage:availableForAllPageTypes" access="global" controller="LightningComponentSortingPagnation">
<!-- call doInit method on component load -->
<aura:handler name="init" value="{!this}" action="{!c.doInit}"/>
<!-- aura attributes to store data -->
<aura:attribute name="listOfAccounts" type="list"/>
<aura:attribute name="paginationList" type="list"/>
<aura:attribute name="currentPageNumber" type="Integer" default="1"/>
<aura:attribute name="pageSize" type="Integer" default="10"/>
<aura:attribute name="totalPages" type="Integer" default="0"/>
<aura:attribute name="pageList" type="List"/>
<aura:attribute name="totalRecords" type="Integer" />
<aura:attribute name="currentPageRecords" type="Integer" />
<!-- sorting data variables -->
<aura:attribute name="columns" type="List"/>
<aura:attribute name="sortedBy" type="String" default="Name"/>
<aura:attribute name="sortedDirection" type="string" default="asc" />
<div class="slds-tabs_card">
<div class="slds-page-header">
<div class="slds-page-header__row">
<div class="slds-page-header__col-title">
<div class="slds-media">
<div class="slds-media__figure">
<span class="slds-icon_container slds-icon-standard-opportunity">
<lightning:icon iconName="standard:event" alternativeText="Event" title="Event" />
</span>
</div>
<div class="slds-media__body">
<div class="slds-page-header__name">
<div class="slds-page-header__name-title">
<h1>
<span>Lightning Component Datatable with Sorting and pagination</span>
<span class="slds-page-header__title slds-truncate" title="TechDicer">TechDicer</span>
</h1>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div> <br/>
<lightning:card title="Account Data with Sorting and Pagination" iconName="standard:account">
<p class="slds-var-p-around_small">
<lightning:layout multipleRows="true" horizontalAlign="center">
<lightning:layoutItem padding="around-small" size="12">
<lightning:datatable keyField="id" data="{!v.paginationList}"
columns="{!v.columns}"
hideCheckboxColumn="true"
onsort="{!c.updateSorting}"
sortedBy="{!v.sortedBy}"
sortedDirection="{!v.sortedDirection}" />
</lightning:layoutItem>
<!-- Pagination Buttons Start -->
<div class="slds-align_absolute-center">
<lightning:button label="First"
iconName="utility:left"
iconPosition="left"
onclick="{!c.onFirst}"
disabled="{! v.currentPageNumber == 1}" />
<lightning:button label="Previous"
disabled="{!v.currentPageNumber == 1}"
onclick="{!c.handlePrevious}"
variant="brand"
iconName="utility:back"
name="previous"/>
<span class="slds-badge slds-badge_lightest"
style="margin-right: 10px;margin-left: 10px;">
Page {!v.currentPageNumber} out of {!v.totalPages}
</span>
<lightning:button label="Next"
disabled="{!v.currentPageNumber == v.totalPages}"
onclick="{!c.handleNext}"
variant="brand"
iconName="utility:forward"
iconPosition="right"
name="next"/>
<lightning:button label="Last"
iconName="utility:right"
iconPosition="right"
onclick="{!c.onLast}"
disabled="{!v.currentPageNumber == v.totalPages}" />
</div>
</lightning:layout>
</p>
</lightning:card>
</aura:component>
LightningComponentSortingController.JS :
({
doInit : function(component, event, helper) {
component.set('v.columns', [
{label: 'Name', fieldName:'Name', sortable:true, type:'text'},
{label: 'Phone', fieldName:'Phone', sortable:true, type:'phone'},
{label: 'Type', fieldName:'Type', sortable:true, type:'text'},
{label: 'Created Date', fieldName: 'CreatedDate',type: 'date', sortable:true,
typeAttributes:{day:'numeric',month:'short',year:'numeric',hour:'2-digit',minute:'2-digit',second:'2-digit',hour12:true}}
]);
helper.fetchAccounts(component, helper);
},
updateSorting: function (cmp, event, helper) {
var fieldName = event.getParam('fieldName');
var sortDirection = event.getParam('sortDirection');
cmp.set("v.sortedBy", fieldName);
cmp.set("v.sortedDirection", sortDirection);
helper.sortData(cmp, fieldName, sortDirection);
},
handleNext: function(component, event, helper){
component.set("v.currentPageNumber", component.get("v.currentPageNumber") + 1);
helper.setPaginateData(component);
},
handlePrevious: function(component, event, helper){
component.set("v.currentPageNumber", component.get("v.currentPageNumber") - 1);
helper.setPaginateData(component);
},
onFirst: function(component, event, helper) {
component.set("v.currentPageNumber", 1);
helper.setPaginateData(component);
},
onLast: function(component, event, helper) {
component.set("v.currentPageNumber", component.get("v.totalPages"));
helper.setPaginateData(component);
},
})
LightningComponentSortingHelper.Js :
({
fetchAccounts : function(component, helper) {
var action = component.get("c.fetchAccountData");
action.setCallback(this, function(response) {
var state = response.getState();
if(state === 'SUCCESS'){
var result = response.getReturnValue();
component.set("v.listOfAccounts", result);
helper.preparePagination(component, result);
}
});
$A.enqueueAction(action);
},
preparePagination: function (component, records) {
let countTotalPage = Math.ceil(records.length / component.get("v.pageSize"));
let totalPage = countTotalPage > 0 ? countTotalPage : 1;
component.set("v.totalPages", totalPage);
component.set("v.currentPageNumber", 1);
component.set("v.totalRecords", records.length);
this.setPaginateData(component);
},
setPaginateData: function(component){
let data = [];
let pageNumber = component.get("v.currentPageNumber");
let pageSize = component.get("v.pageSize");
let accountData = component.get('v.listOfAccounts');
let currentPageCount = 0;
let x = (pageNumber - 1) * pageSize;
currentPageCount = x;
for (; x < (pageNumber) * pageSize; x++){
if (accountData[x]) {
data.push(accountData[x]);
currentPageCount++;
}
}
component.set("v.paginationList", data);
component.set("v.currentPageRecords", currentPageCount);
},
sortData: function (cmp, fieldName, sortDirection) {
var fname = fieldName;
var data = cmp.get("v.listOfAccounts");
var reverse = sortDirection !== 'asc';
data.sort(this.sortBy(fieldName, reverse))
cmp.set("v.listOfAccounts", data);
this.setPaginateData(cmp);
},
sortBy: function (field, reverse) {
var key = function(x) {return x[field]};
reverse = !reverse ? 1 : -1;
return function (a, b) {
return a = key(a), b = key(b), reverse * ((a > b) - (b > a));
}
},
})
Output :
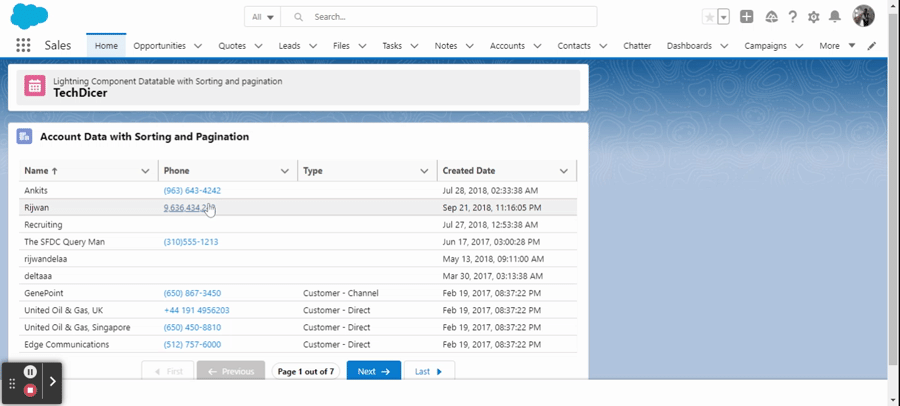
1 comment
Thank you !! it Helped so much.