Hello friends, today we will discuss Rest Resource Apex Class in Salesforce. We create the Apex Rest Resource class to expose the salesforce objects with the third party and perform operations. In this way, we hit the Salesforce custom API by a third-party app.
Also, check this: Add Hyperlink Column in LWC Datatable
Key Highlights :
- Apex REST API accepts data in XML and JSON format.
- We also need to create a site in Salesforce for a third-party app.
- Class is annotated with @RestResource(urlMapping=’/customNamspace/*’). So the third-party URL will be https://instance.salesforce.com/services/apexrest/customNamspace/.
- https://instance.salesforce.com/ This is your salesforce site URL which you can easily find out when you log in to your org.
- We can create, delete, and update Salesforce records.
- We use workbench for testing.
Syntax :
@RestResource(urlMapping='/techdicer/*') global with sharing class TehcdicerRestResource{ @HttpGet global static void getData(){} //Reads or retrieves records. @HttpPost global static void postData(){} //Creates records. @HttpPut global static void putData(){}//Typically used to update existing records or create records. @HttpDelete global static void deleteData(){}//use for Deletes records. }
Process & Code :
@RestResource(urlMapping='/techdicer/*') global with sharing class TechdicerRestResource { //here we delete contact @HttpDelete global static String doDelete() { RestRequest req = RestContext.request; RestResponse res = RestContext.response; String contactId = req.requestURI.substring(req.requestURI.lastIndexOf('/') + 1); Contact con = [SELECT Id FROM Contact WHERE Id = :contactId]; delete con; return json.serialize('Contact deleted successfully!!'); } //here we get contact @HttpGet global static Contact doGet() { RestRequest req = RestContext.request; RestResponse res = RestContext.response; String contactId = req.requestURI.substring(req.requestURI.lastIndexOf('/') + 1); Contact con = [SELECT Id, Name, Phone, Email FROM Contact WHERE Id = :contactId]; return con; } //here we create record and upload files @httpPost global static account doPost() { List<ContentVersion> contentVersionList = new List<ContentVersion>(); List<ContentDocumentLink> contentDocumentLinkList = new List<ContentDocumentLink>(); RequestData reqData = parse(RestContext.Request.requestBody.toString()); system.debug('reqData==> ' + reqData); //fetch files from json response List<cls_files> files = (List<cls_files>)reqData.files; //get recordId Account account = new Account(); account.Name = (String)reqData.name; insert account; Id accId = account.Id; if(String.isNotBlank(accId) && !files.isEmpty()){ for(cls_files file : files){ ContentVersion cv = new ContentVersion(); cv.PathOnClient = file.name + '.' + file.type;//File name with extension cv.Title = file.name;//Name of the file cv.VersionData = EncodingUtil.base64Decode(file.binary); contentVersionList.add(cv); } if(!contentVersionList.isEmpty()){ insert contentVersionList; for(ContentVersion cv : [SELECT ContentDocumentId FROM ContentVersion WHERE Id IN:contentVersionList]){ ContentDocumentLink cdl = new ContentDocumentLink(); cdl.ContentDocumentId = cv.ContentDocumentId; cdl.LinkedEntityId = accId; contentDocumentLinkList.add(cdl); } insert contentDocumentLinkList; } } return account; } public class RequestData { public String name; public cls_files[] files; } class cls_files { public String name; //test public String binary; //binartdata public String type; //pdf } public static RequestData parse(String json){ return (RequestData) System.JSON.deserialize(json, RequestData.class); } }
@isTest public class TechdicerRestResourceTest { @testSetup static void dataSetup() { // Create test accounts List<Contact> testconts = new List<Contact>(); for(Integer i=0;i<2;i++) { testconts.add(new Contact(Lastname = 'TestAcct'+i)); } insert testconts; } public static testMethod void unitTest1(){ Contact con = [SELECT Id FROM Contact LIMIT 1]; RestRequest req = new RestRequest(); RestResponse res = new RestResponse(); req.requestURI = '/services/apexrest/techdicer/' + con.Id; req.httpMethod = 'Delete'; //req.addHeader('Content-Type', 'application/json'); //req.requestBody = Blob.valueof('{ "recordId" : "' + ld.Id + '", "files":[{ "name" :"test", "binary" : "iVBORw0KGgoAAAANSUhEUgAAABgAA", "type" : "pdf" }] }'); RestContext.request = req; RestContext.response = res; Test.startTest(); TechdicerRestResource.doDelete(); Test.stopTest(); } public static testMethod void unitTest2(){ Contact con = [SELECT Id FROM Contact LIMIT 1]; RestRequest req = new RestRequest(); RestResponse res = new RestResponse(); req.requestURI = '/services/apexrest/techdicer/' + con.Id; req.httpMethod = 'Get'; //req.addHeader('Content-Type', 'application/json'); //req.requestBody = Blob.valueof('{ "recordId" : "' + ld.Id + '", "files":[{ "name" :"test", "binary" : "iVBORw0KGgoAAAANSUhEUgAAABgAA", "type" : "pdf" }] }'); RestContext.request = req; RestContext.response = res; Test.startTest(); TechdicerRestResource.doGet(); Test.stopTest(); } public static testMethod void unitTest3(){ RestRequest req = new RestRequest(); RestResponse res = new RestResponse(); req.requestURI = '/services/apexrest/techdicer'; req.httpMethod = 'Post'; req.addHeader('Content-Type', 'application/json'); req.requestBody = Blob.valueof('{ "name" : "TestAccount", "files":[{ "name" :"test", "binary" : "iVBORw0KGgoAAAANSUhEUgAAABgAA", "type" : "pdf" }] }'); RestContext.request = req; RestContext.response = res; Test.startTest(); TechdicerRestResource.doPost(); Test.stopTest(); } }
Output :
Login workbench > After logging in, select utilities | REST Explorer. check below images for call rest resource class.
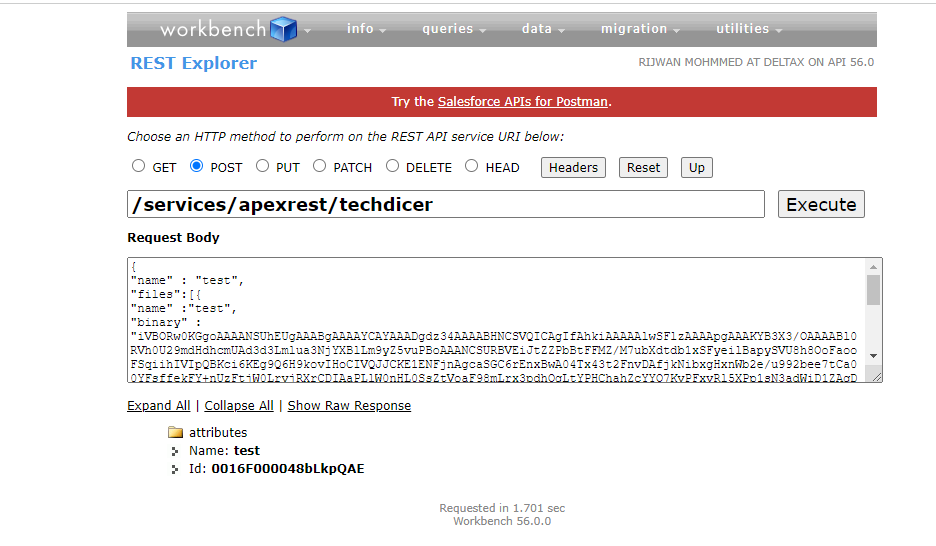
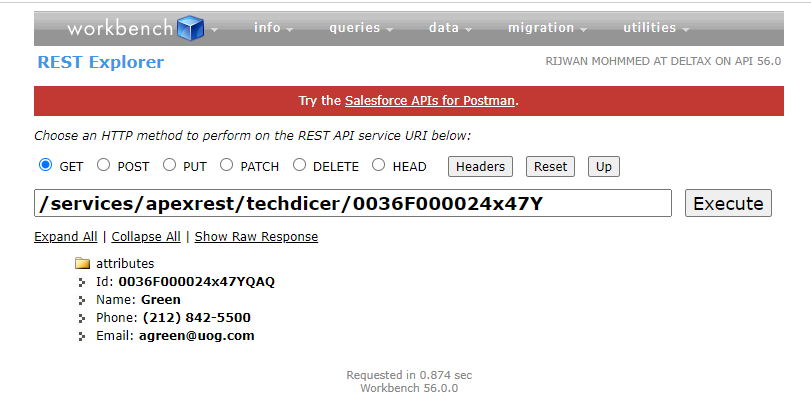
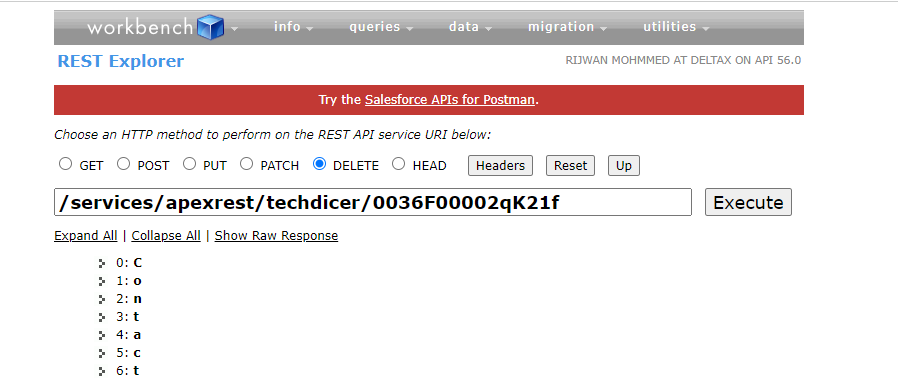
Reference :
What’s your Reaction?
+1
1
+1
2
+1
+1
+1
4
+1