Hello friends, today we are going to discuss Retrieve Schedule Jobs in Apex LWC. Here we will create an LWC component and show the Schedule Jobs of the salesforce org with proper details. The AsyncApexJob object has all info of async jobs.
Also, check this: Rest Resource Apex Class in Salesforce
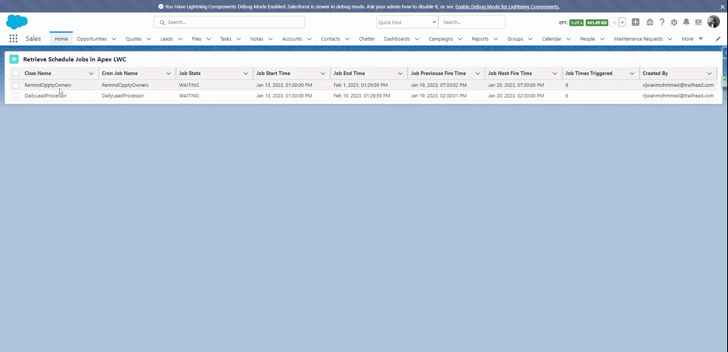
Key Highlights :
- Can check all scheduled jobs.
- Query the AsyncApexJob with the Cron details.
- CronTrigger contains schedule information for a scheduled job.
Code :
AsyncJobs.cls :
public class AsyncJobs { @AuraEnabled(cacheable=true) public static List<AsyncWrapper> fetchAsyncJobs(){ //query the AsyncApexJobs List <AsyncApexJob> asyncApexJobList = [select Id, ApexClass.Name, Status, CronTrigger.CronJobDetail.Name, CronTrigger.CronExpression, CronTrigger.State, CronTrigger.StartTime, CronTrigger.EndTime, CronTrigger.PreviousFireTime, CronTrigger.NextFireTime, CronTrigger.TimesTriggered, CronTrigger.CreatedBy.Username, CronTrigger.CreatedDate, CronTrigger.LastModifiedBy.Username from AsyncApexJob where JobType in ('ScheduledApex') ORDER BY CronTrigger.TimesTriggered DESC NULLS LAST ]; List<AsyncWrapper> asyncWrapperList = new List<AsyncWrapper>(); //itrate the AsyncApexJobs if any for (AsyncApexJob job : asyncApexJobList) { AsyncWrapper wrapper = new AsyncWrapper(); wrapper.className = job.ApexClass.Name; wrapper.cronJobName = job.CronTrigger.CronJobDetail.Name; wrapper.cronExpression = job.CronTrigger.CronExpression; wrapper.jobState = job.CronTrigger.State; wrapper.jobStartTime = job.CronTrigger.StartTime; wrapper.jobEndTime = job.CronTrigger.EndTime; wrapper.jobPreviouseFireTime = job.CronTrigger.PreviousFireTime; wrapper.jobNextFireTime = job.CronTrigger.NextFireTime; wrapper.jobTimesTriggered = job.CronTrigger.TimesTriggered; wrapper.createdByUserName = job.CronTrigger.CreatedBy.Username; asyncWrapperList.add(wrapper); } return asyncWrapperList; } public class AsyncWrapper{ @AuraEnabled public String className; @AuraEnabled public String cronJobName; @AuraEnabled public String cronExpression; @AuraEnabled public String jobState; @AuraEnabled public Datetime jobStartTime; @AuraEnabled public Datetime jobEndTime; @AuraEnabled public Datetime jobPreviouseFireTime; @AuraEnabled public Datetime jobNextFireTime; @AuraEnabled public Integer jobTimesTriggered; @AuraEnabled public String createdByUserName; public AsyncWrapper(){} } }
lWCAsyncJobs.HTML :
<template> <lightning-card title="Retrieve Schedule Jobs in Apex LWC" icon-name="standard:macros"> <div class="slds-p-horizontal_small"> <div if:true={data}> <lightning-datatable data={data} columns={columns} key-field="Id"></lightning-datatable> </div> </div> </lightning-card> </template>
lWCAsyncJobs.JS:
import { LightningElement, wire } from 'lwc'; import fetchAsyncJobs from '@salesforce/apex/AsyncJobs.fetchAsyncJobs'; const columns = [ { label: 'Class Name', fieldName: 'className', type: 'text', }, { label: 'Cron Job Name', fieldName: 'cronJobName', type: 'text', }, { label: 'Job State', fieldName: 'jobState', type: 'text' }, { label: 'Job Start Time', fieldName: 'jobStartTime', type: 'date', typeAttributes: { day: 'numeric', month: 'short', year: 'numeric', hour: '2-digit', minute: '2-digit', second: '2-digit', hour12: true } }, { label: 'Job End Time', fieldName: 'jobEndTime', type: 'date', typeAttributes: { day: 'numeric', month: 'short', year: 'numeric', hour: '2-digit', minute: '2-digit', second: '2-digit', hour12: true } }, { label: 'Job Previouse Fire Time', fieldName: 'jobPreviouseFireTime', type: 'date', typeAttributes: { day: 'numeric', month: 'short', year: 'numeric', hour: '2-digit', minute: '2-digit', second: '2-digit', hour12: true } }, { label: 'Job Next Fire Time', fieldName: 'jobNextFireTime', type: 'date', typeAttributes: { day: 'numeric', month: 'short', year: 'numeric', hour: '2-digit', minute: '2-digit', second: '2-digit', hour12: true } }, { label: 'Job Times Triggered', fieldName: 'jobTimesTriggered' }, { label: 'Created By', fieldName: 'createdByUserName', type: 'text' } ]; export default class LWCAsyncJobs extends LightningElement { data = []; columns = columns; @wire(fetchAsyncJobs) wireAccounts({ error, data }) { if (data) { console.log(data); this.data = data; this.error = undefined; } else if (error) { this.error = error; } } }
lWCAsyncJobs.js-meta.xml :
<?xml version="1.0"?> <LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata"> <apiVersion>55.0</apiVersion> <isExposed>true</isExposed> <targets> <target>lightning__HomePage</target> </targets> </LightningComponentBundle>
Output :
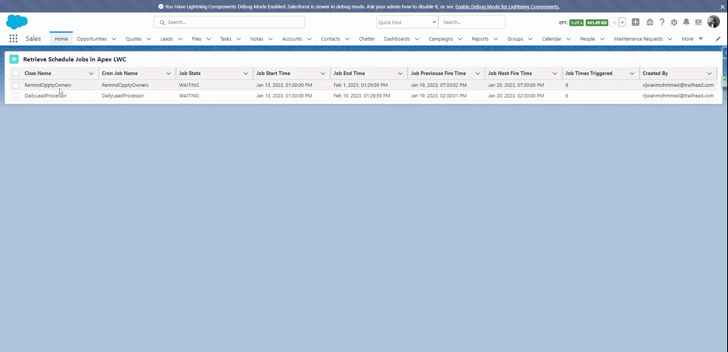
Reference :
What’s your Reaction?
+1
+1
+1
+1
+1
+1