Hello friends, today we are going to discuss How to set Picklist in LWC Datatable Inline Edit Salesforce. In LWC Datatable there are limited field types and the Picklist type is not there. But not to worry We will do this by creating a custom type Datatable and will create the Picklist type field.
Inline editing is a game-changer, and now, you can enhance it further with picklist fields. Editing picklist values directly within a Datatable boosts efficiency and user satisfaction.
Also, check this: Chain Wire methods in LWC
Key Highlights :
- Create a Picklist type field in Datatable
- We can edit this field
- We can show/hide this picklist by the pen icon.
- Update the data.
- Picklist options fetch dynamically for LWC Datatable.
- Efficiency: No more navigating away to edit picklists. Users can update them instantly within the table.
- User Experience: Enhance user satisfaction by reducing the steps needed for data updates.
- Accuracy: Prevent errors by ensuring users select from valid picklist values.
🚀 Empower Your LWCs: By incorporating picklist inline editing in Datatables, you’re unlocking a smoother and more intuitive data management experience. Whether it’s managing leads, contacts, or any records with picklist fields, this feature raises your LWC development to the next level.
Give it a shot and see the transformation in your user interactions! 🌐🎉
Process & Code :
AccountDataController.cls :
public class AccountDataController {
@AuraEnabled (cacheable=true)
public static List<Account> fetchAccounts(){
return [SELECT Id, Name, Type, Phone
FROM Account WHERE Type !='' LIMIT 10];
}
}
lWCCustomDatatableType.HTML : We create a custom type Datatable here which extends standard LWC Datatable. Also, create an extra HTML file picklistColumn.html, pickliststatic.html so we can put the Picklist Combobox and its static value here. Below image of the structure
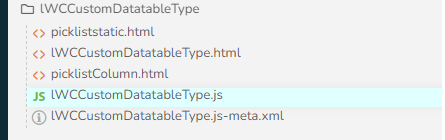
<template>
</template>
picklistColumn.html :
<template> <lightning-combobox name="picklist" data-inputable="true" label={typeAttributes.label} value={editedValue} placeholder={typeAttributes.placeholder} options={typeAttributes.options} variant='label-hidden' dropdown-alignment="auto"></lightning-combobox> </template>
pickliststatic.html :
<template> <span class="slds-truncate" title={value}>{value}</span> </template>
lWCCustomDatatableType.JS:
import LightningDatatable from 'lightning/datatable';
import picklistColumn from './picklistColumn.html';
import pickliststatic from './pickliststatic.html'
export default class LWCCustomDatatableType extends LightningDatatable {
static customTypes = {
picklistColumn: {
template: pickliststatic,
editTemplate: picklistColumn,
standardCellLayout: true,
typeAttributes: ['label', 'placeholder', 'options', 'value', 'context', 'variant','name']
}
};
}
Now we will create the last final LWC component
lWCDatatableWithPicklist.html :
<template>
<!-- header -->
<div class="slds-tabs_card">
<div class="slds-page-header">
<div class="slds-page-header__row">
<div class="slds-page-header__col-title">
<div class="slds-media">
<div class="slds-media__figure">
<span class="slds-icon_container slds-icon-standard-opportunity">
<lightning-icon icon-name="standard:recipe" alternative-text="recipe" title="recipe"></lightning-icon>
</span>
</div>
<div class="slds-media__body">
<div class="slds-page-header__name">
<div class="slds-page-header__name-title">
<h1>
<span>PickList In LWC Inline Datatable Edit</span>
<span class="slds-page-header__title slds-truncate" title="Recently Viewed">TechDicer</span>
</h1>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div> <br/>
<!-- /header -->
<!-- create folder card -->
<lightning-card variant="Narrow" title="PickList In LWC Inline Datatable Edit" icon-name="standard:folder" class="cardSpinner">
<!-- loader -->
<div if:true={showSpinner}>
<lightning-spinner
alternative-text="Loading..." variant="brand">
</lightning-spinner>
</div>
<!-----/loader-------->
<div class="slds-var-p-around_small">
<template if:true={data}>
<c-l-w-c-custom-datatable-type
key-field="Id"
data={data}
columns={columns}
onvalueselect={handleSelection}
draft-values={draftValues}
oncellchange={handleCellChange}
onsave={handleSave}
oncancel={handleCancel}
hide-checkbox-column>
</c-l-w-c-custom-datatable-type>
</template>
</div>
</lightning-card>
</template>
lWCDatatableWithPicklist.JS:
import { LightningElement, track, wire } from 'lwc';
import fetchAccounts from '@salesforce/apex/AccountDataController.fetchAccounts';
import ACCOUNT_OBJECT from '@salesforce/schema/Account';
import TYPE_FIELD from '@salesforce/schema/Account.Type';
import { updateRecord } from 'lightning/uiRecordApi';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
import { refreshApex } from '@salesforce/apex';
import { getPicklistValues, getObjectInfo } from 'lightning/uiObjectInfoApi';
const columns = [
{ label: 'Name', fieldName: 'Name', editable: true },
{ label: 'Phone', fieldName: 'Phone', type: 'phone', editable: true },
{
label: 'Type', fieldName: 'Type', type: 'picklistColumn', editable: true, typeAttributes: {
placeholder: 'Choose Type', options: { fieldName: 'pickListOptions' },
value: { fieldName: 'Type' }, // default value for picklist,
context: { fieldName: 'Id' } // binding account Id with context variable to be returned back
}
}
]
export default class CustomDatatableDemo extends LightningElement {
columns = columns;
showSpinner = false;
@track data = [];
@track accountData;
@track draftValues = [];
lastSavedData = [];
@track pickListOptions;
@wire(getObjectInfo, { objectApiName: ACCOUNT_OBJECT })
objectInfo;
//fetch picklist options
@wire(getPicklistValues, {
recordTypeId: "$objectInfo.data.defaultRecordTypeId",
fieldApiName: TYPE_FIELD
})
wirePickList({ error, data }) {
if (data) {
this.pickListOptions = data.values;
} else if (error) {
console.log(error);
}
}
//here I pass picklist option so that this wire method call after above method
@wire(fetchAccounts, { pickList: '$pickListOptions' })
accountData(result) {
this.accountData = result;
if (result.data) {
this.data = JSON.parse(JSON.stringify(result.data));
this.data.forEach(ele => {
ele.pickListOptions = this.pickListOptions;
})
this.lastSavedData = JSON.parse(JSON.stringify(this.data));
} else if (result.error) {
this.data = undefined;
}
};
updateDataValues(updateItem) {
let copyData = JSON.parse(JSON.stringify(this.data));
copyData.forEach(item => {
if (item.Id === updateItem.Id) {
for (let field in updateItem) {
item[field] = updateItem[field];
}
}
});
//write changes back to original data
this.data = [...copyData];
}
updateDraftValues(updateItem) {
let draftValueChanged = false;
let copyDraftValues = [...this.draftValues];
//store changed value to do operations
//on save. This will enable inline editing &
//show standard cancel & save button
copyDraftValues.forEach(item => {
if (item.Id === updateItem.Id) {
for (let field in updateItem) {
item[field] = updateItem[field];
}
draftValueChanged = true;
}
});
if (draftValueChanged) {
this.draftValues = [...copyDraftValues];
} else {
this.draftValues = [...copyDraftValues, updateItem];
}
}
//handler to handle cell changes & update values in draft values
handleCellChange(event) {
//this.updateDraftValues(event.detail.draftValues[0]);
let draftValues = event.detail.draftValues;
draftValues.forEach(ele=>{
this.updateDraftValues(ele);
})
}
handleSave(event) {
this.showSpinner = true;
this.saveDraftValues = this.draftValues;
const recordInputs = this.saveDraftValues.slice().map(draft => {
const fields = Object.assign({}, draft);
return { fields };
});
// Updateing the records using the UiRecordAPi
const promises = recordInputs.map(recordInput => updateRecord(recordInput));
Promise.all(promises).then(res => {
this.showToast('Success', 'Records Updated Successfully!', 'success', 'dismissable');
this.draftValues = [];
return this.refresh();
}).catch(error => {
console.log(error);
this.showToast('Error', 'An Error Occured!!', 'error', 'dismissable');
}).finally(() => {
this.draftValues = [];
this.showSpinner = false;
});
}
handleCancel(event) {
//remove draftValues & revert data changes
this.data = JSON.parse(JSON.stringify(this.lastSavedData));
this.draftValues = [];
}
showToast(title, message, variant, mode) {
const evt = new ShowToastEvent({
title: title,
message: message,
variant: variant,
mode: mode
});
this.dispatchEvent(evt);
}
// This function is used to refresh the table once data updated
async refresh() {
await refreshApex(this.accountData);
}
}
lWCDatatableWithPicklist.css :
.cardSpinner{
position: relative;;
}
147 comments
Hii Rijwan the dropdown is not opening like that for me ,even when I used the same code.Can you please help
Can you please share your file structure.
Hi Rijwan the dropdown works perfect for me when i put de LWC in a tab but it doesn’t when I put it on a record page, do you know why? Thank you!!
Can you please share a screen shot or video link.
Also, check the position of the dropdown list.
I have updated the CSS file which is the static resource LWCDatatablePicklist. Can you please check now.
Hi Rijwan,
first thank for this code :). I have an error when i try to save new picklist value i receive
‘Field Type does not exist ‘
lwc002_EditInvoice.js:1 {Id: ‘a16Aa0000000PCxIAM’, Type: ‘Commit’}
t @ aura_prod.js:1
lwc002_EditInvoice.js:1 {status: 400, body: {…}, headers: {…}, ok: false, statusText: ‘Bad Request’, …}body: {message: ‘Field Type does not exist.’, statusCode: 400, errorCode: ‘POST_BODY_PARSE_ERROR’}errorType: “fetchResponse”headers: {}ok: falsestatus: 400statusText: “Bad Request”[[Prototype]]: Object
Could you help me
check getPicklistValues , I think you are putting field which is not there in Org
I tried your code, but options are undefined in the pickerColumn. I have checked wire is working correctly.
here is the column object
{
label: ‘Day Part’, fieldName: ‘label’, type: ‘picklistColumn’, editable: false, typeAttributes: {
placeholder: ‘Choose Type’,
options: { fieldName: ‘pickListOptions’ },
value: { fieldName: ‘label’ }, // default value for picklist,
context: { fieldName: ‘index’ } // binding account Id with context variable to be returned back
}
}
pickListOptions is getting values or not in JS
Hi Rijwan. Thank you! Your code works perfectly for me. Data in all columns are edited and saved correctly. Except for picklist column. When I edit the picklist value, it doesn’t save(An error occured!!). What could be wrong?
What was the error?
Can you please check Line no 108 in CustomDatatableDemo.JS
When I change the picklist value and tried to save it, I got the nest error:
“Error! An error ocurred!!”.
You’re right. Thank you!
can you show multi inline picklist with checked box?
yes we can use multi-select picklist.
data table save and cancel button at the bottom of datatable is not getting open when we change the value in combo box
Can you please send me a screenshot, As per my understanding it is working fine. If you have time I will post a new blog regarding this in simple steps at the end of this week.
Hi Rijwan,
I able to load datatable but while clicking on picklist, Type picklist values is not loading.
Hi Saloni,
please check below code and check console
//fetch picklist options
@wire(getPicklistValues, {
recordTypeId: “$objectInfo.data.defaultRecordTypeId”,
fieldApiName: TYPE_FIELD
})
wirePickList({ error, data }) {
if (data) {
this.pickListOptions = data.values;
} else if (error) {
console.log(error);
}
}
HI,
I want to use picklist for multiple columns.
Can you suggest how I can set picklistOptions for different columns.
Hi MOHIT CHAUHAN
Just create other one only field type should be type: ‘picklistColumn’
I am unable to show different values in options for different picklist, because I am fetching columns dynamically from apex method with the help of metadata using field sets.
picklistColumns=YEAR_FIELD;
@wire (getMarketingPlanColumns, {currentMarketingPlanCountry: ‘$Country’})
marketingPlanColumns(result) {
if(result.data) {
this.columns = JSON.parse(JSON.stringify(result.data));
this.columns.push({
type: ‘action’,
typeAttributes:{rowActions: action}
});
this.columns.forEach(column => {
if(column.type == “PICKLIST”){
// @wire(getPicklistValues, {recordTypeId: ‘$recordTypeId’, fieldApiName: COUNTRY_FIELD})
// wirePickList({ error, data }) {
// if (data) {
// this.pickListOptions = data.values;
// console.log(this.pickListOptions);
// } else if (error) {
// console.log(‘error ‘+error);
// }
// };
column.typeAttributes = {
placeholder: ‘Choose Type’,
options: { fieldName: ‘pickListOptions’ },
value: { fieldName: ‘column.fieldName’ }, // default value for picklist,
context: { fieldName: ‘Id’ } // binding account Id with context variable to be returned back
}
}
})
// console.log(this.columns);
}
};
The issue in your JSON put type: ‘picklistColumn’ on columns where you want to the picklist. This is possible.
Hi Rijwan,
I am not getting where you mentioned ‘Just create other one only field type should be type: ‘picklistColumn’’ Can you please provide more detail on this one. I am able to get one picklist only, for other it is blank, even though wire method to get picklist has values.
@Isha, Please setup a meeting by click book a meeting button on my website in right side
Hi Rijan
Not able to get picklist value in UI but it showing correcly in console.log
const columns = [
{ label: ‘Claim ID’, fieldName: ‘ClaimID__c’ },
{ label: ‘Member Name’, fieldName: ‘MH_MemberName__c’, type: ‘text’ },
{ label: ‘DOS From’, fieldName: ‘MH_DOSFrom__c’, type: ‘text’ },
{ label: ‘DOS To’, fieldName: ‘MH_DOSTo__c’, type: ‘text’ },
{ label: ‘Status’, fieldName: ‘MH_Status__c’, type: ‘text’ },
{label: ‘Denial Reason’, fieldName: ‘MH_Denial_Reason__c’, type: ‘picklistColumn’, editable: true, typeAttributes: {
placeholder: ‘Choose Type’, options: { fieldName: ‘pickListOptions’ },
value: { fieldName: ‘MH_Denial_Reason__c’ }, // default value for picklist,
context: { fieldName: ‘Id’ } // binding account Id with context variable to be returned back
}
}
];
export default class BasicDatatable extends LightningElement {
@api recordId;
@track recdata;
columns = columns;
@track pickListOptions;
draftValues = [];
@wire(getObjectInfo, { objectApiName: CASE_CLAIM })
objectInfo;
@wire(getPicklistValues, {recordTypeId: ‘$objectInfo.data.defaultRecordTypeId’,fieldApiName: DENIAL_REASON })
getdenialreason({ error, data }) {
if (data) {
console.log(‘datapick’);
console.log(data);
this.pickListOptions = data.values;
console.log(‘this.pickListOptions’);
console.log(JSON.stringify(this.pickListOptions));
} else if (error) {
console.log(‘pickListOptionserror’);
console.log(error);
}
}
Hi @Balaji,
Have you tried my code with type picklist
Yes but I have used your code LWCCustomDatatable and parent component is different
import { LightningElement,api,wire,track } from ‘lwc’;
import getdenialcashclaim from ‘@salesforce/apex/mh_caseclaimcontroller.getcashclaim’;
import { updateRecord } from ‘lightning/uiRecordApi’;
import { ShowToastEvent } from ‘lightning/platformShowToastEvent’;
import { refreshApex } from ‘@salesforce/apex’;
import { getPicklistValues, getObjectInfo } from ‘lightning/uiObjectInfoApi’;
import CASE_CLAIM from ‘@salesforce/schema/MH_CaseClaims__c’;
import DENIAL_REASON from ‘@salesforce/schema/MH_CaseClaims__c.MH_Denial_Reason__c’;
//import pickListValueDynamically from ‘@salesforce/apex/mh_caseclaimcontroller.pickListValueDynamically’;
const columns = [
{ label: ‘Claim ID’, fieldName: ‘ClaimID__c’ },
{ label: ‘Member Name’, fieldName: ‘MH_MemberName__c’, type: ‘text’ },
{ label: ‘DOS From’, fieldName: ‘MH_DOSFrom__c’, type: ‘text’ },
{ label: ‘DOS To’, fieldName: ‘MH_DOSTo__c’, type: ‘text’ },
{ label: ‘Status’, fieldName: ‘MH_Status__c’, type: ‘text’ },
{
label: ‘Denial Reason’, fieldName: ‘MH_Denial_Reason__c’, type: ‘picklistColumn’, editable: true, typeAttributes: {
label:{fieldName: ‘pickListOptions’},
placeholder: ‘Select Reason’, options: { fieldName: ‘pickListOptions’ },
value: { fieldName: ‘MH_Denial_Reason__c’ }, // default value for picklist,
context: { fieldName: ‘Id’ } // binding account Id with context variable to be returned back
}
}
];
export default class BasicDatatable extends LightningElement {
@api recordId;
@track recdata;
columns = columns;
@track pickListOptions;
draftValues = [];
@wire(getObjectInfo, { objectApiName: CASE_CLAIM })
objectInfo;
@wire(getPicklistValues, {recordTypeId: ‘$objectInfo.data.defaultRecordTypeId’,fieldApiName: DENIAL_REASON })
getdenialreason({ error, data }) {
if (data) {
console.log(‘datapick’);
console.log(data);
this.pickListOptions = data.values;
console.log(‘this.pickListOptions’);
console.log(JSON.stringify(this.pickListOptions));
} else if (error) {
console.log(‘pickListOptionserror’);
console.log(error);
}
}
/* @wire(pickListValueDynamically, {customObjInfo: {‘sobjectType’ : ‘MH_CaseClaims__c’},
selectPicklistApi: ‘MH_Denial_Reason__c’})
getdenialreason({ error, data }) {
if (data) {
console.log(‘this.pickListOptions’);
console.log(data);
this.pickListOptions = data;
} else if (error) {
console.log(‘pickListOptionserror’);
console.log(error);
}
};*/
@wire(getdenialcashclaim,{recordId:’$recordId’})
getclaimrecord({error, data}){
if(data){
console.log(‘data’);
console.log(data);
this.recdata = data;
this.error = undefined;
}else{
console.log(‘error’);
this.error = error;
this.recdata = undefined;
}
}
}
*********************************************
Hi @Balaji, I think you missed something, Can you please check you HTML part .
Hi Rijwan,
getting this error -> Invalid reference Order.Fsys_BillingType__c of type sobjectField in file fsys_PendingBillingReport.js: Source
Does your functionality work on custom fields??? I am getting this error on this line-> import OWNER_FIELD from ‘@salesforce/schema/Order.Fsys_BillingType__c’;
I was writing the code on Lightning studio(beta)
HI Shiam Singh Rawat, This also work for custom objects
Issue in Lightning studio(beta), you have to checked the checkbox in bottom one then deploy it will works
Hi Rijwan,
Thank you so much for paving the way here. I’ve tried to use your strategy in my component, but I’m getting an error in the console when I click on the pencil icon to edit the picklist field. “Cannot read properties of null (reading ‘value’).
And there is also another error: Editable custom types must define an editTemplate that includes an element with attribute data-inputable set to “true”.
Not sure if the two errors are related. I’ve checked my picklistColumn.html and it definitely has data-inputable=”true” as a property.
Any ideas?
Hi @Jim,
Did you check all components deployed properly
Hi Rijwan
Can you tell me How to add upload file button in data table & how to add three picklist in single data table. Out of these three picklist one picklist is dependent picklist.
Hi Gopal, the upload file button can be added like row action add in LWC data table.
also, multiple picklists can be added but dependent picklists, no idea about this.
Thank you Rijwan
missing root template tag error in picklistColumn.html
check all components code again.
hi Rijwan,
Sorry I am new LWC so can you confrim me are we creating two LWCs here right?
Bcoz I am confused with your file structure,
Thank you
Shallet
yupp
Thank you so much!. I have done it but I am not able to see the picklist values in the list. can you help me?
how to filter the child records in above code can you please modify that
@Kari please check this link for filter data
https://techdicer.com/filter-search-in-lwc-lightning-datatable/
Hi Rijwan,
I asked for a call. But you did not join. Please let me know what is the best time to do so. I need your help.
Can you please arrange one more meeting today? Sorry I missed this
Hi Rijwan,
I have sent another invite for tomorrow. Please have a look.
Hi Rijwan,
How to fetch different two picklist fields with different values ?
Ex : Field A has values Yes, No
Field B has values Right, Wrong
On UI , its rendering Yes, No for both picklist fields. What did I miss here ? Could you please advise ?
Hi Rijwan,
Can we connect today at 5pm for quick call? Unable to fetch picklist fields with different values. Please assist.
Thank you!
Sai Mohan
Hi @Sai, Please schedule meeting by click this button in right side of my website >>Book Meeting For Free
Thanks Rijwan you solved last 2 weeks issue thank you so much Great
@Rijwan
Can you help me for multi select picklist
Let me first check for multi-select picklist
Hi Rizwan I had some confusion can you send me entire code and how many components we need to build to get this ui please let me know and pls send the whole code I had some requirements in my project
There are two components only.
Hi @Rizwan,
will it work for more than one picklist field like ‘type’ and ‘industry’ picklist field in datatable?
Yupp , it will work for multiple Picklists
Hi, Thanks for the article.
I am facing a problem How can I add params in the @wire?
I’ve changed the apex this way.
public static List fetchAccounts(Id scheduledClassId)
Now how can I add an extra param in the wire?
@wire(fetchAccounts, {pickList: ‘$pickListOptions’ })
@wire(fetchAccounts, {scheduledClassId : ‘test’, pickList: ‘$pickListOptions’ })
hi Rijwan,
I am getting below message intermittently ….can you help me please?
message
:
“An error occurred while trying to update the record. Please try again.”
output
:
errors
:
Array(1)
0
:
{constituentField: null, duplicateRecordError: null, errorCode: ‘UNABLE_TO_LOCK_ROW’, field: null, fieldLabel: null, …}
Thank you
Hi Rizwan, I am getting an Error While Saving the Record
Can you please share the error
The picklist shows the API name, how to show the label for it?
Hi @aahna Singh
In picklistColumn.html file
I used variant=’label-hidden’ in combobox so If you want to show label remove this variant.
hi, tried that. Did not work, still shows the API name of the picklist values on the datatable.
What about pickliststatic.html? Any changes need to be made there?
Please setup a meeting by click book a meeting button on my website in right side
I requested a call. But you did not join. Please let me know what is the best time to do so.
I am sorry for the inconvenience. You can schedule the call on sunday
I scheduled a call for Sunday at 12 PM for your convenience, but you didn’t participate in that one either. Please let me know a time and date that work for you.
I already reschedule it
“I have the same question. How can I deal with it?
Hi Rizwan, can you post this scenario-related code?
custom LWC picklist component with a search option as in the Standard Salesforce.
Pass the object that exists in the org & on selecting any object from the dropdown, display the records in the table with the inline edit option.
Hi @Rajeswari, I can but it will take some time.
hello i have declared my column like this : {
label: ‘Résiliation’, fieldName: ‘Resiliation__c’, type: ‘picklistColumn’, editable: true, typeAttributes: {
placeholder: ”, options: { fieldName: ‘pickListOptions’ },
value: { fieldName: ‘Resiliation__c’ }, // default value for picklist,
context: { fieldName: ‘Id’ } // binding account Id with context variable to be returned back
}
}
And for the options ;
@wire(getObjectInfo, { objectApiName: DOSSIER_REVALO_OBJECT })
objectInfo;
//fetch picklist options
@wire(getPicklistValues, {
recordTypeId: “$objectInfo.data.defaultRecordTypeId”,
fieldApiName: RESILIATION_FIELD
})
wirePickList({ error, data }) {
if (data) {
this.pickListOptions = data.values;
} else if (error) {
console.log(error);
}
console.log(this.pickListOptions);
} ant the console log returuns value but in the front i get blank options
Check line number 55 in my JS code.
Hey Rijwan,
What to do if I want to reduce my picklist column size I am decreasing the size of lightning combo-box but its only decreasing leaving a blank space behind it. How to decrease the size of whole component (here the whole picklist)
Schedule a call on sunday
Upon fetching picklist values it automatically transformed array to object
I don`t know why did it do it
In wire:
[{“label”:”Hot”,”value”:”Hot”},{“label”:”Warm”,”value”:”Warm”},{“label”:”Cold”,”value”:”Cold”}]
In picklist template
{“0”:{“label”:”Hot”,”value”:”Hot”},”1″:{“label”:”Warm”,”value”:”Warm”},”2″:{“label”:”Cold”,”value”:”Cold”}}
Please check the code , may be you are doing casting.
Let me know If you want We can connect on call on click Book a meeting on my website.
Hello, my datatable is working great except one of my picklist values has a special character ‘N/A’. The values show correctly upon selecting N/A but once saved it saves it as the api value of ‘n_a’. How can I update to have it save as the label value?
You can book a meeting on my website
Hello Rijwan, I have implemented the above code.
Now when i click edit, It loading as a picklist but its only showing the placeholder not the entire picklist options.
Also i have passed the picklist option from Apex using schema instead of getting in LWC. Here a JSON format for the same.
“typeAttributes”: {
“editable”: true,
“objectApiName”: “CCM_Master”,
“fieldApiName”: “CCM_Business_Code__c”,
“placeholder”: “Select an option”,
“options”: [
{“label”: “CFR”, “value”: “CFR”},
{“label”: “CIF”, “value”: “CIF”},
{“label”: “CIP”, “value”: “CIP”},
{“label”: “CPT”, “value”: “CPT”},
{“label”: “DAP”, “value”: “DAP”},
{“label”: “DDP”, “value”: “DDP”},
{“label”: “DPU”, “value”: “DPU”},
{“label”: “FAS”, “value”: “FAS”},
{“label”: “FCA”, “value”: “FCA”},
{“label”: “FOB”, “value”: “FOB”}
]
}
Can you please check console log
Hello Rijwan, when check console log using debug mode i am not getting any error.
Can we check of the options value has been passed to child from parent or not? As the placeholder value is passing but not the options when click edit.
This is how i am getting the value in my parent component.
connectedCallback() {
loadStyle(this, modal);
console.log(‘Id: ‘ + this.RecordId);
getdata({ recordId: this.RecordId})
.then((response) => {
if (response) {
console.log(response);
this.tables = response.map((table) => ({
id: table.id,
name: table.name,
data: table.dataList ? table.dataList.map((record) => ({
…record,
deleteRow: ‘Delete’,
isNew: false,
})) : [],
columns: table.columns.map((column) => {
if (column.type === ‘picklistColumn’) {
return {
…column,
type: ‘picklistColumn’,
editable: true,
typeAttributes: {
editable: true,
objectApiName: ‘CCM_Business_Code__c’,
fieldApiName: column.fieldName,
placeholder: ‘Select an option’,
options: column.picklistOptions.map((option) => ({
label: option,
value: option,
})),
},
};
} else {
return {
…column,
editable: true,
};
}
}),
draftValues: [],
}));
console.log(‘tables’ + this.tables);
console.log(‘Agr’ + JSON.stringify(this.tables));
}
})
.catch((error) => {
console.error(‘Error retrieving data’, error);
})
.finally(() => {
this.isLoading = false;
});
}
And on the HTML Side
>
Hello Rijwan, i just tried something, look like it issue with inline edit. When i click the inline edit option, It not rendering the dropdown.
When i assigned “template: picklistColumn” During the initial load it getting all the picklist options. Any reason why it is happening ?
static customTypes = {
picklistColumn: {
template: picklistColumn,
editTemplate: picklistColumn,
standardCellLayout: true,
typeAttributes: [‘label’, ‘placeholder’, ‘options’, ‘value’, ‘context’, ‘variant’, ‘name’]
}
};
I have the same issue:
When i click the inline edit option, It not rendering the dropdown.
When i assigned “template: picklistColumn” During the initial load it getting all the picklist options.
How did you solve this?
Please book a meeting I can help you
The this is how i have defined on HTML Side:
>
>
Hi Rijwan, what about multi picklist.
Could you find any solution for multi-picklist?
Yupp, https://techdicer.com/multiselect-picklist-in-lwc-datatable-inline-edit/
Thank you for your help.
The reference code seems to work as expected.
I hope this information helps many people.
There is a package called List Editor that can do something very similar.
(https://appexchange.salesforce.com/appxListingDetail?listingId=a0N3u00000PFXi6EAH&tab=r)
However, List Editor has two disadvantages: I would appreciate it if you could point me to some code that can solve it someday.
・When batch editing with the pencil mark on the upper right, numeric keypad input is not accepted for currency type.
・When batch editing with the pencil mark on the upper right, after searching for the reference item, you can not select the item without clicking with the mouse.
Hello,
How do i do this for multiple picklist fields? I need to add another one.
Thanks,
Joscelyn
This is only for Single select Picklist.
Rijwan,
I need to know how to pass multiple picklists in this wire function call:
//here I pass picklist option so that this wire method call after above method
@wire(fetchAttendees, { pickList: ‘$pickListOptions’, id: ‘$recordId’})
attendeeData(result) {
Do I add another param pickList2? I tried booking meeting but it won’t let me.
Tried replicating your code in my developer org, the table renders as expected but picklist values do not render when I click the inline-edit. In the console log, the ‘pickListOptions’ variable was populated after the @wire render.
Hi DJ, did you copy full code because I also passing picklistoption in next wire method so it will call after this (chaining wire method)
I used below code to get dynamic picklist values
import { LightningElement, wire} from ‘lwc’;
import { getPicklistValues, getObjectInfo } from ‘lightning/uiObjectInfoApi’;
import TMT_OBJECT from ‘@salesforce/schema/Account’;
import TMTTYPE_FIELD from ‘@salesforce/schema/Account.Type’;
const columns = [
{ label: ‘Name’, fieldName: ‘Name’, editable: true },
{ label: ‘Phone’, fieldName: ‘Phone’, type: ‘phone’, editable: true },
{
label: ‘Type’, fieldName: ‘Type’, type: ‘picklistColumn’, editable: true, typeAttributes: {
placeholder: ‘Choose Type’, options: { fieldName: ‘pickListOptions’ },
value: { fieldName: ” }, // default value for picklist,
//context: { fieldName: ‘Id’ } // binding account Id with context variable to be returned back
}
}
]
export default class TestLwcDataTable extends LightningElement {
pickListOptions = [];
data = [{Name: ‘UserA’, Phone: ‘12345’, Type:”}];
columns = columns;
@wire(getObjectInfo, { objectApiName: TMT_OBJECT })objectInfo;
@wire(getPicklistValues, {
recordTypeId: ‘$objectInfo.data.defaultRecordTypeId’,
fieldApiName: TMTTYPE_FIELD
})wirePickList({ error, data }) {
if (data) {
console.log(‘data===>’,data);
const dataVal = Object.values(data.values);
console.log(‘pickList===>’,dataVal);
this.pickListOptions = dataVal;
console.log(‘pickListOptions===>’,this.pickListOptions);
} else if (error) {
console.log(error);
}
}
}
Did you see my comment?
Rijwan,
I need to know how to pass multiple picklists in this wire function call:
//here I pass picklist option so that this wire method call after above method
@wire(fetchAttendees, { pickList: ‘$pickListOptions’, id: ‘$recordId’})
attendeeData(result) {
Do I add another param pickList2? I tried booking meeting but it won’t let me.
Can you add another picklist?
If so, how do I do it?
HI @Joscelyn
please book a meeting on below link
I can help you on call
https://topmate.io/rijwan_mohmmed
Hi Rijwan,
Is it possible to manage multi select picklist in LWC Datatable?
Hi Huseyin,
I will try this today.
https://techdicer.com/multiselect-picklist-in-lwc-datatable-inline-edit/
Could you find any solution for multi-picklist?
Hi, me again. We had a discussion last week and you helped me add a second picklist. Is there a way to make it a dependent picklist in the datatable?
Thanks,
Joscelyn
Hi @Joscelyn Stonis
It may be possible but it will be very complex
hey how can we add two custom picklist in same datatable
We can add. Please schedule a meeting by click book a meeting
How to show pencil icon always to the right side of column
How to show pencil icon always to the right side of column, don’t want to show on hover only
Hi , Can we make a pick list field mandatory?
Yes we can make this.
Thanks for the blog. I m trying the snippet code I m facing issues related to save button there is a slight change in my design. I used the component in flow and flow binds the tabledata. after click save I m unable to refresh the tabledata. Can you suggest any other alternative how to refresh the table data. In my case wire refresh method may not work as table data passed as api property from flow.
You can imperative apex class method for get data in datatable
Hi Rijwan,
I am using your code with a custom object and custom field but I am not getting the values in the combobox in the datatable, values are coming perfectly in the logs. Let me know if it is possible for you to connect. Thank you
Hi SS, Please schedule a meeting by click below link
https://topmate.io/rijwan_mohmmed
Hello Rizwan, I would like to thank you, it worked perfectly for my needs mainly because with this logic, I can mark multiple rows and replicate them instantly as if it were a standard column. I’m new and I ended up learning more because I didn’t know what dynamic and reactive variables were and I learned it by trying to find out the reason for the comment on line 48. I really liked your work.
Thank you again!
call wire method in Chaining
https://techdicer.com/chain-wire-methods-in-lwc/
hi rijiwan, can this code runs on denpendent picklist values? i’ve tried but it seemed now working, thank u!
Hi @theo, I don’t think so, But I will post a blog on this as soon as possible
Hi, Thank you for the code. But the picklist always hides and i have to scroll to see the values.
Is there any way to display the picklist above the lwc?
Hi Rizwan, I am getting everything correct except the picklist options are not coming up. Please help. I have populate the picklist attributes in the incoming results from apex and it is working correctly. But when clicking on the drop down nothing comes.
Can you please schedule a booking of meeting so I can resolve your issue. Booking button already there on my website .
If I wanted to be able to feed the picklist with fixed options, I tried this:
@track pickListOptions = [
{ label: ‘Option 1’, value: ‘1’ },
{ label: ‘Option 2’, value: ‘2’ },
{ label: ‘Option 3’, value: ‘3’ }
];
But it does not work. Does anyone know how I should make it work? I’m new to Salesforce
Hi Juan,
Can you please schedule a booking of meeting so I can resolve your issue. Booking button already there on my website .
Hi Rijwan
Thanks for the blog, I tired using the same however, the picklist values are not visible over UI, they came is white card blocks(which matches the number of picklist options) when I open the drop down.(Console get the value of picklist options).
I tired to connect with you on 6th Jan 24 at 1PM but you didn’t joined.
Could you please help me on this.
Hi Rijwan,
How to fetch different two picklist fields with different values ?
Ex : Field A has values Yes, No
Field B has values Right, Wrong
On UI , its rendering Yes, No for both picklist fields. What did I miss here ? Could you please advise ?
Hi @Priyanka,
Can you please schedule a booking for meeting for your issue
https://topmate.io/rijwan_mohmmed
Hey Rijwan, I tried your code not getting the save and cancel button on edit of picklist values. Could you please help me out what could be the possible reason for this.
Hi @Shivam
Can you please schedule a booking for meeting for your issue
https://topmate.io/rijwan_mohmmed
HI Rijwan ,
This code works fine for me for one column picklist fields.
How to make this work for 10 columns ?
Please advice
Hi Chaya, Can you please explain this more
Picklist options are visible if we have one field with picklist datatype as per the code you have provided.
I have a requirement to work on 10 picklist field from account object.
I added the same code replicated for 1 more field.
But same picklist option is displaying for both fields.
Could you please suggest how to fix this issue ?
book a meeting https://topmate.io/rijwan_mohmmed, I will help you
Hello
ele.pickListOptions = this.pickListOptions;
in this snippet, ele.pickListOptions is never populated eventhough I see data in this.pickListOptions;.
What should the data look like in this.pickListOptions;
Hello
When trying the new picklist is never populated. I just see ‘Choose Type’
this.data.forEach(ele => {
ele.pickListOptions = this.pickListOptions;
})
Even though there in this.pickListOptions it does not update ele.pickListOptions
Thoughts? also, can this replace a picklist field that is being populated on load. So if there is a value selected for then record will it show that value?
Thanks
Hi @Phuc, Please book a meeting on below link https://topmate.io/rijwan_mohmmed
Currently the picklist API name is showing in the non-editable format, can we show the label instead of the picklist?
Hi Rijwan,
Thank you very much for sharing the code. I tried to implement this in my opportunity object related list opportunity products. I have created a button that opens the lightning data table. Somehow, even after trying several times I couldn’t change my type from text to picklist field.
Also, I have width as controlling field for length picklist field.
Please find my main component js code below,
import { LightningElement, wire, api, track } from ‘lwc’;
import { NavigationMixin } from ‘lightning/navigation’;
import { ShowToastEvent } from ‘lightning/platformShowToastEvent’;
import { refreshApex } from ‘@salesforce/apex’;
import getOpportunityProducts from ‘@salesforce/apex/OpportunityProductController.getOpportunityProducts’;
import updateOpportunityProducts from ‘@salesforce/apex/OpportunityProductController.updateOpportunityProduct’;
import deleteOpportunityProduct from ‘@salesforce/apex/OpportunityProductController.deleteOpportunityProduct’;
import OPPORTUNITYLINEITEM_OBJECT from ‘@salesforce/schema/OpportunityLineItem’;
import { getPicklistValues, getObjectInfo } from ‘lightning/uiObjectInfoApi’;
import UNIT_OF_MEASURE_FIELD from ‘@salesforce/schema/OpportunityLineItem.Unit_of_Measure__c’;
import WIDTH_FIELD from ‘@salesforce/schema/OpportunityLineItem.Width__c’;
import LENGTH_FIELD from ‘@salesforce/schema/OpportunityLineItem.Length__c’;
export default class OppProductEdit extends NavigationMixin(LightningElement) {
@api recordId;
@track opportunityProducts = [];
@track draftValues = [];
wiredOpportunityProductsResult;
unitOfMeasureOptions;
widthOptions;
lengthOptions;
columns = [
{ label: ‘Product ID’, fieldName: ‘Product2Id’, type: ‘text’ },
{ label: ‘Quantity’, fieldName: ‘Quantity’, type: ‘number’, editable: true },
{ label: ‘Unit of Measure’, fieldName: ‘Unit_of_Measure__c’, type: ‘picklist’,
typeAttributes: { placeholder: ‘Choose…’, options: { fieldName: ‘unitOfMeasureOptions’ },
value: { fieldName: ‘Unit_of_Measure__c’ }, context: { fieldName: ‘Id’ } }, editable: true },
{ label: ‘Unit Price’, fieldName: ‘UnitPrice’, type: ‘currency’, editable: true },
{ label: ‘Description’, fieldName: ‘Description’, type: ‘text’, editable: true },
{ label: ‘Width’, fieldName: ‘Width__c’, type: ‘picklist’,
typeAttributes: { placeholder: ‘Choose…’, options: { fieldName: ‘widthOptions’ },
value: { fieldName: ‘Width__c’ }, context: { fieldName: ‘Id’ } }, editable: true },
{ label: ‘Length’, fieldName: ‘Length__c’, type: ‘picklist’, typeAttributes: { placeholder: ‘Choose…’, options: { fieldName: ‘lengthOptions’ }, value: { fieldName: ‘Length__c’ }, context: { fieldName: ‘Id’ } }, editable: true },
{ label: ‘Product Code’, fieldName: ‘ProductCode’, type: ‘text’ },
{ label: ‘Total Price’, fieldName: ‘TotalPrice’, type: ‘currency’ },
{
type: ‘button-icon’,
typeAttributes: {
iconName: ‘utility:delete’,
name: ‘delete’,
title: ‘Delete’,
variant: ‘bare’,
alternativeText: ‘Delete’
}
}
];
@wire(getObjectInfo, { objectApiName: OPPORTUNITYLINEITEM_OBJECT })
objectInfo;
//Fetch picklist options
@wire(getPicklistValues, { recordTypeId: ‘$objectInfo.data.defaultRecordTypeId’, fieldApiName: UNIT_OF_MEASURE_FIELD })
unitOfMeasureValues({ error, data }) {
if (data) this.unitOfMeasureOptions = data.values;
else if (error) this.showToast(‘Error’, ‘Failed to load Unit of Measure options’, ‘error’);
}
@wire(getPicklistValues, { recordTypeId: ‘$objectInfo.data.defaultRecordTypeId’, fieldApiName: WIDTH_FIELD })
widthValues({ error, data }) {
if (data) this.widthOptions = data.values;
else if (error) this.showToast(‘Error’, ‘Failed to load Width options’, ‘error’);
}
@wire(getPicklistValues, { recordTypeId: ‘$objectInfo.data.defaultRecordTypeId’, fieldApiName: LENGTH_FIELD })
lengthValues({ error, data }) {
if (data) this.lengthOptions = data.values;
else if (error) this.showToast(‘Error’, ‘Failed to load Length options’, ‘error’);
}
@wire(getOpportunityProducts, {picklist: ‘$picklistOptions’})
OpportunityLineItemData(result) {
this.OpportunityLineItemData = result;
if(result.data){
this.data = JSON.parse(JSON.stringify(result.data));
this.data.forEach(ele => {
ele.pickListOptions = this.pickListOptions;
})
this.lastSavedData = JSON.parse(JSON.stringify(this.data));
} else if (result.error){
this.data = undefined;
}
}
updateDataValues(updateItem) {
let copyData = JSON.parse(JSON.stringify(this.data));
copyData.forEach(item => {
if (item.Id === updateItem.Id) {
for (let field in updateItem) {
item[field] = updateItem[field];
}
}
});
//write changes back to original data
this.data = […copyData];
}
updateDraftValues(updateItem) {
let draftValueChanged = false;
let copyDraftValues = […this.draftValues];
//store changed value to do operations
//on save. This will enable inline editing &
//show standard cancel & save button
copyDraftValues.forEach(item => {
if (item.Id === updateItem.Id) {
for (let field in updateItem) {
item[field] = updateItem[field];
}
draftValueChanged = true;
}
});
if (draftValueChanged) {
this.draftValues = […copyDraftValues];
} else {
this.draftValues = […copyDraftValues, updateItem];
}
}
//handler to handle cell changes & update values in draft values
handleCellChange(event) {
//this.updateDraftValues(event.detail.draftValues[0]);
let draftValues = event.detail.draftValues;
draftValues.forEach(ele=>{
this.updateDraftValues(ele);
})
}
@wire(getOpportunityProducts, { opportunityId: ‘$recordId’ })
wiredOpportunityProducts(result) {
this.wiredOpportunityProductsResult = result;
if (result.data) {
this.opportunityProducts = result.data;
} else if (result.error) {
this.showToast(‘Error’, result.error.body.message, ‘error’);
}
}
handleSave(event) {
const recordsInputs = event.detail.draftValues.slice().map(draft => {
const fields = { …draft, Id: draft.Id };
return { fields };
});
const promises = recordsInputs.map(recordInput =>
updateOpportunityProducts({ data: recordInput })
);
Promise.all(promises)
.then(() => {
this.showToast(‘Success’, ‘Products updated successfully.’, ‘success’);
this.draftValues = [];
return refreshApex(this.wiredOpportunityProductsResult);
})
.catch(error => {
this.showToast(‘Error’, error.body.message, ‘error’);
});
}
handleCancel() {
console.log(‘Navigating back to record page:’, this.recordId);
// Standard navigation
this[NavigationMixin.Navigate]({
type: ‘standard__recordPage’,
attributes: {
recordId: this.recordId,
objectApiName: ‘Opportunity’,
actionName: ‘view’
}
});
// Fallback navigation
setTimeout(() => {
window.location.href = ‘/’ + this.recordId;
}, 500); // Delay to allow standard navigation to process first
}
handleRowAction(event) {
const actionName = event.detail.action.name;
const row = event.detail.row;
if (actionName === ‘delete’) {
this.deleteProduct(row.Id);
}
}
deleteProduct(productId) {
deleteOpportunityProduct({ productId })
.then(() => {
this.showToast(‘Success’, ‘Product deleted successfully’, ‘success’);
return refreshApex(this.wiredOpportunityProductsResult);
})
.catch(error => {
this.showToast(‘Error’, error.body.message, ‘error’);
});
}
showToast(title, message, variant) {
this.dispatchEvent(
new ShowToastEvent({
title,
message,
variant
})
);
}
}
Hi @Divya, Please book a meeting on below link https://topmate.io/rijwan_mohmmed