Hello friends, today we will discuss Upload CSV and Create Records in LWC. It becomes complicated to upload CSV files to create records if the records and column values contain quotes. This method will create account records when a CSV file is uploaded.
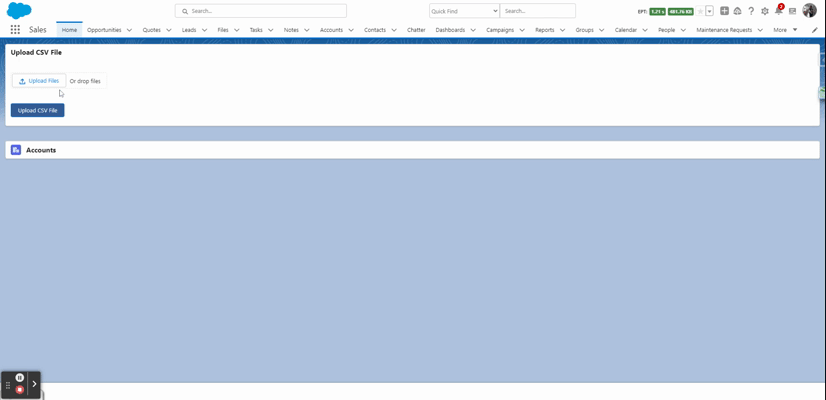
Also, check this: Fetch Records By LIST VIEW Id In Apex
Key Highlights :
- When it comes to handling large volumes of data, the ability to import and create records from CSV files can save valuable time and effort.
- We use the
lightning-file-upload
component provided by Salesforce. - Once the CSV file is uploaded, we can use JavaScript and Apex to process the file and extract the data.
Code :
Step 1: Create a CSV file that contains records of the Account object. Link of CSV File
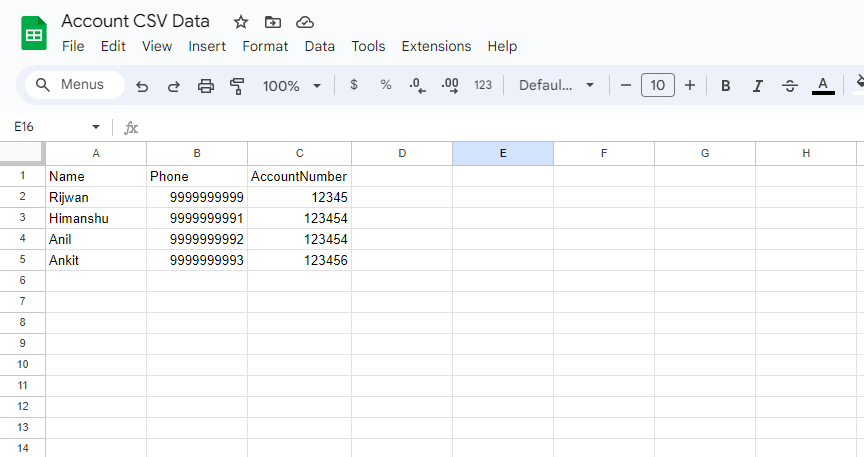
Step 2: Create an Apex Class named as 'AccountDataCreateByCSV'. This class is used for create account records by CSV file. AccountDataCreateByCSV.cls :
/** * @description : This class is used for create account records by CSV file. * @coverage : Test.cls | @CC100% * @author : techdicerkeeplearning@gmail.com * @group : Techdicer * @last modified on : 05-27-2023 * @last modified by : techdicerkeeplearning@gmail.com * Modifications Log * Ver Date Author Modification * 1.0 05-27-2023 techdicerkeeplearning@gmail.com Initial Version | Story Number | @CC100% **/ public class AccountDataCreateByCSV { /** * @description create account records by CSV file. * @author techdicerkeeplearning@gmail.com | 05-27-2023 | Story Number * @param base64Data * @return List<Account> returns the provided list of accounts **/ @AuraEnabled public static List<Account> createAccountRecords(String base64Data) { String data = JSON.deserializeUntyped(base64Data).toString(); List<Account> accList = new List<Account>(); List<String> lstCSVLines = data.split('\n'); for(Integer i = 1; i < lstCSVLines.size(); i++){ Account acc = new Account(); String csvLine = lstCSVLines[i]; String prevLine = csvLine; Integer startIndex; Integer endIndex; while(csvLine.indexOf('"') > -1){ if(startIndex == null){ startIndex = csvLine.indexOf('"'); csvLine = csvLine.substring(0, startIndex) + ':quotes:' + csvLine.substring(startIndex+1, csvLine.length()); }else{ if(endIndex == null){ endIndex = csvLine.indexOf('"'); csvLine = csvLine.substring(0, endIndex) + ':quotes:' + csvLine.substring(endIndex+1, csvLine.length()); } } if(startIndex != null && endIndex != null){ String sub = csvLine.substring(startIndex, endIndex); sub = sub.replaceAll(',', ':comma:'); csvLine = csvLine.substring(0, startIndex) + sub + csvLine.substring(endIndex, csvLine.length()); startIndex = null; endIndex = null; } } List<String> csvRowData = new List<String>(); for(String column : csvLine.split(',')){ column = column.replaceAll(':quotes:', '').replaceAll(':comma:', ','); csvRowData.add(column); } acc.Name = csvRowData[0]; acc.Phone = csvRowData[1]; acc.AccountNumber = csvRowData[2]; accList.add(acc); } if(!accList.isEmpty()){ insert accList; } return accList; } }
Step 3:
@isTest public class AccountDataCreateByCSV { static String str = 'Name,Phone,AccountNumber\n Rijwan,9999999999,10101\n Rijwan,9999999999,10101'; static testmethod void testfileupload(){ Test.startTest(); Blob csvFileBody = Blob.valueOf(str); String s = EncodingUtil.base64Encode(csvFileBody); AccountDataCreateByCSV.createAccountRecords(s); Test.stopTest(); } }
Step 4: Create a Lightning Web Component named cSVFileUploaderLWC.
cSVFileUploaderLWC.html :
<template> <!-- loader --> <div if:true={showSpinner}> <lightning-spinner variant="brand" alternative-text="Uploading......"> </lightning-spinner> </div> <!-------------> <lightning-card title="Upload CSV File"> <div class="slds-p-around_small"> <div> <lightning-input label="" name="file uploader" onchange={handleFilesChange} type="file" multiple> </lightning-input> </div> <br/> <div class="slds-text-body_small slds-text-color_error">{fileName} </div><br/> <div> <lightning-button class="slds-m-top--medium" label={UploadFile} onclick={handleSave} variant="brand" disabled={isTrue}></lightning-button> </div> </div> </lightning-card> <br/><br/> <lightning-card title="Accounts" icon-name="standard:account"> <div style="width: auto;"> <template if:true={data}> <lightning-datatable data={data} columns={columns} key-field="id"> </lightning-datatable> </template> </div> </lightning-card> </template>
cSVFileUploaderLWC.JS:
import { LightningElement, track, api } from 'lwc'; import createAccountRecords from '@salesforce/apex/AccountDataCreateByCSV.createAccountRecords'; import { ShowToastEvent } from 'lightning/platformShowToastEvent'; const columns = [ { label: 'Name', fieldName: 'Name' }, { label: 'Phone', fieldName: 'Phone'}, { label: 'Account Number', fieldName: 'AccountNumber' } ]; export default class CSVFileUploaderLWC extends LightningElement { @api recordid; @track columns = columns; @track data; @track fileName = ''; @track UploadFile = 'Upload CSV File'; @track showSpinner = false; @track isTrue = false; selectedRecords; filesUploaded = []; file; fileContents; fileReader; content; MAX_FILE_SIZE = 1500000; handleFilesChange(event) { if (event.target.files.length > 0) { this.filesUploaded = event.target.files; this.fileName = event.target.files[0].name; } } handleSave() { if (this.filesUploaded.length > 0) { this.uploadHelper(); } else { this.fileName = 'Please select a CSV file to upload!!'; } } uploadHelper() { this.file = this.filesUploaded[0]; if (this.file.size > this.MAX_FILE_SIZE) { console.log('File Size is to long'); return; } this.showSpinner = true; this.fileReader = new FileReader(); this.fileReader.onloadend = (() => { this.fileContents = this.fileReader.result; this.saveToFile(); }); this.fileReader.readAsText(this.file); } saveToFile() { createAccountRecords({ base64Data: JSON.stringify(this.fileContents), cdbId: this.recordid }) .then(result => { console.log(result); this.data = result; this.fileName = this.fileName + ' - Uploaded Successfully'; this.isTrue = false; this.showSpinner = false; this.showToast('Success', this.file.name + ' - Uploaded Successfully!!!', 'success', 'dismissable'); }) .catch(error => { console.log(error); this.showToast('Error while uploading File', error.body.message, 'error', 'dismissable'); }); } showToast(title, message, variant, mode) { const evt = new ShowToastEvent({ title: title, message: message, variant: variant, mode: mode }); this.dispatchEvent(evt); } }
cSVFileUploaderLWC.JS-xml:
<?xml version="1.0"?> <LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata"> <apiVersion>57.0</apiVersion> <isExposed>true</isExposed> <targets> <target>lightning__HomePage</target> </targets> </LightningComponentBundle>
Output :
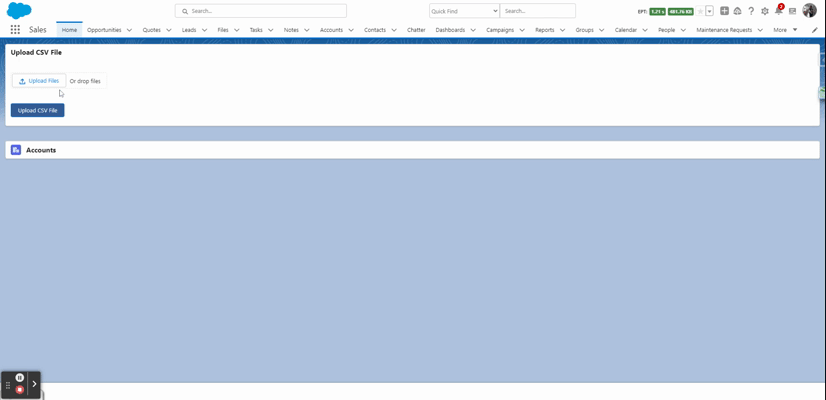
Reference :
What’s your Reaction?
+1
2
+1
+1
+1
+1
5
+1
8 comments
Hi RIJWAN,
Similar Way Create a code When Order Information Uploading Create Account,Order,Order Item.
Make Neccessary Changes .
Can U Share The Same on my Email…
Thank You Advance..
Thanks for such great article, If you can put the Test Class code as well it would be highly appreciated.
Hi @GG,
Please check now.
code is not not getting covered 100% can u help me with code coverage .
How many records can we create?? I have did this using bulk api… I want to create progress bar as well how many records inserted. How can I achieve this??
https://topmate.io/rijwan_mohmmed
Hi Yogesh,
Please schedule a meeting by click on my website , there is a button. We can discuss further
Hi, RIJWAN.
When I try to upload a large file, I get the following errors: “Too many DML rows: 10001” and “File Size is too long”. Could you please tell me how to fix this?
Can you please schedule a call