Hello friends, today we are going to discuss Lifecycle Hooks in LWC. A lifecycle hook is a callback method triggered at a specific phase of a component instance’s lifecycle. In Lightning web components have a lifecycle that is managed by the framework. The framework creates components, inserts them into the DOM, renders them, and removes them from the DOM. Additionally, it monitors changes to component properties. Although these lifecycle hooks are not required, it is possible for components to call them.
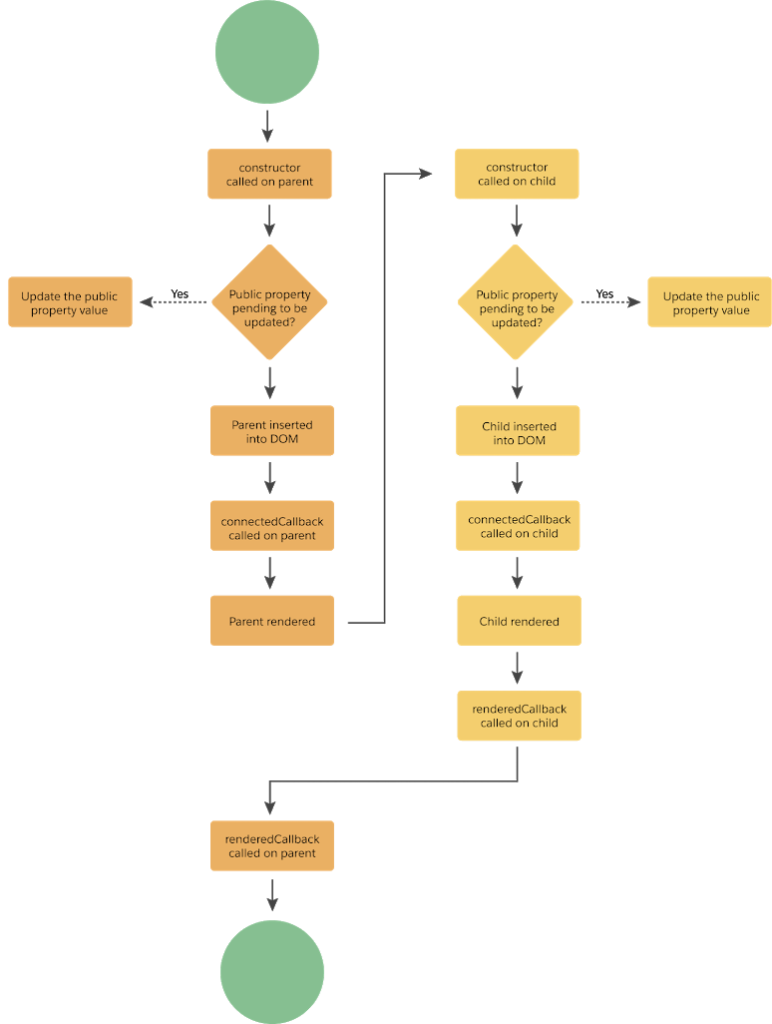
Also, check this: Apex defined variable in Salesforce Flow
Key Highlights :
- Constructor: When a component instance is created, constructor() is called.
- ConnectedCallback: when a component is inserted into the DOM.
- Render: this will not count in Life cycle hooks but will be use for render templates.
- RenderedCallback: Fires when a component is rendered on the DOM.
- DisconnectedCallback: Fires when a component is removed from the DOM.
- ErrorCallback: Fires in case of any error during a lifecycle hook or event
Code :
1. Constructor():
Called when the component is created. This hook flows from parent to child, meaning it fires in the parent first. You can’t access child elements because they don’t exist yet. Properties aren’t passed yet, either. Properties are assigned to the component after construction and before the connectedCallback()
hook.
import { LightningElement } from 'lwc'; export default class LWCExample1 extends LightningElement { constructor() { super(); this.classList.add('new-class'); } }
2. ConnectedCallback() :
Called when the element is inserted into a document. This hook flows from parent to child. You can’t access child elements because they don’t exist yet.
Use connectedCallback()
to interact with a component’s environment. For example, use it to:
- Establish communication with the current document or container and coordinate behavior with the environment.
- Perform initialization tasks, such as fetching data, setting up caches, or listening for events
- Subscribe and Unsubscribe from a Message Channel.
The connectedCallback()
the hook is invoked with the initial properties passed to the component. If a component derives its internal state from the properties, it’s better to write the logic in a setter than in connectedCallback()
. For example, if you remove an element and then insert it into another position, such as when you reorder a list, the hook fires several times. If you want code to run one time, write code to prevent it from running twice.
import { LightningElement } from 'lwc'; export default class LWCExample1 extends LightningElement { constructor() { super(); this.classList.add('new-class'); } connectedCallback(){ console.log('calling connected callback); } }
3. Render() :
Render() Invoked after the execution of the connectedCallback() method. This hook is used to override the standard rendering functionality in Lightning web components & to update the UI.
- Flows from parent component to child component
- The rendering process can be controlled by conditionally rendering the template on the basis of certain conditions or criteria
- This hook is not technically a lifecycle hook. It is a protected method on the LightningElement class
import { LightningElement } from 'lwc'; import templateOne from './templateOne.html'; import templateTwo from './templateTwo.html'; export default class MiscMultipleTemplates extends LightningElement { showTemplateOne = true; render() { return this.showTemplateOne ? templateOne : templateTwo; } switchTemplate(){ this.showTemplateOne = !this.showTemplateOne; } }
4. RenderedCallback() :
Called after every render of the component. This lifecycle hook is specific to Lightning Web Components, it isn’t from the HTML custom elements specification. This hook flows from child to parent. A component is rerendered when the value of a property changes and that property is used either directly in a component template or indirectly in the getter of a property that is used in a template.
Use renderedCallback()
to interact with a component’s UI. For example, use it to:
- Compute node sizing
- Perform tasks not covered by our template declarative syntax, such as add a listener for a non-standard event from a component’s child
Updating the state of your component in renderedCallback()
can cause an infinite loop. For example:
- Don’t update a wire adapter configuration object property in
renderedCallback()
. See Understand the Wire Service. - Don’t update a public property or field in
renderedCallback()
.
import { LightningElement } from 'lwc'; export default class Boundary extends LightningElement { isRendered = false; renderedCallback() { if(!this.isRendered){ this.isRendered = true; } } }
5. DisconnectedCallback() :
Called when the element is removed from a document. This hook flows from parent to child. Use disconnectedCallback()
to clean up work done in the connectedCallback()
, like purging caches or removing event listeners.
import { LightningElement } from 'lwc'; export default class LWCExample1 extends LightningElement { disconnectedCallback() { console.log('called disconnected call back); } }
6. ErrorCallback(error, stack) :
Called when a descendant component throws an error. The error
argument is a JavaScript native error object and the stack the argument is a string. This lifecycle hook is specific to Lightning Web Components, it isn’t from the HTML custom elements specification.
The method works like a JavaScript catch{}
block for components that throw errors in their lifecycle hooks or in their event handlers declared in an HTML template. It’s important to note that an error boundary component catches errors only from its children, and not from itself.
<!-- boundary.html --> <template> <template lwc:if={error}> <error-view error={error} info={stack}></error-view> </template> <template lwc:elseif={error}> <healthy-view></healthy-view> </template> </template>
// boundary.js import { LightningElement } from 'lwc'; export default class Boundary extends LightningElement { error; stack; errorCallback(error, stack) { this.error = error; } }
Output :
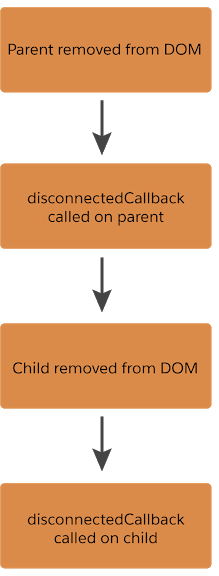
Reference :
- Lifecycle Hooks