Hello folk, today we are going to discuss Salesforce Spring ’23 Release Highlights. Here we will cover mostly developer-side updates.
1. HTTPCallout Builder in Flow: HTTP Callout pulls data from an external system into Flow Builder without using code. You can set up direct integrations as needed without having to work with a developer or call a middleware tool, such as Mulesoft. After you configure the HTTP callout action in a flow, Flow Builder auto-generates an external service registration, an invocable action, and Apex-defined types. You can then use the data output of the API request as input within Flow Builder and across Salesforce. This is in Beta currently.
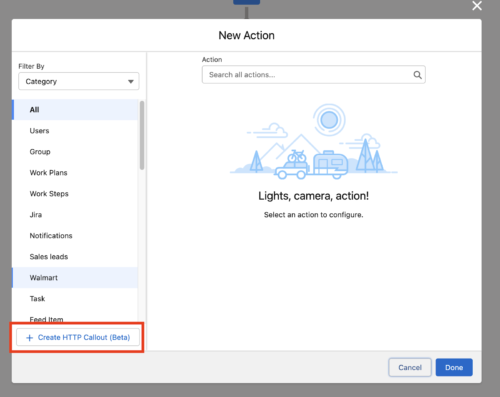
2. Query DOM Elements with Refs: Now you can use refs to access elements in shadow DOM and light DOM. Refs locate DOM elements without a selector and only query elements contained in a specified template. Previously, you could only use querySelector()
to locate specific DOM elements.
Eg. :
<template> <!-- here we giv name of ref --> <div lwc:ref="myDiv"></div> </template>
export default class extends LightningElement { renderedCallback() { //here we query the div element like we do by querySelector() console.log(this.refs.myDiv); } }
3. Use the Improved Conditional Directives: Now we can use lwc:if
, lwc:elseif
, and lwc:else
conditional directives in place of the legacy if:true
and if:else
directives. The legacy if:true
and if:else
directives are no longer recommended as we intend to deprecate and remove these directives in the future.
<!-- conditionalExample.html --> <template> <template lwc:if={isTemplateOne}> This is template one. </template> <template lwc:else> This is template two. </template> <!-- example.html --> <template> <template lwc:if={expression1}> Statement 1 </template> <template lwc:elseif={expression2}> Statement 2 </template> <template lwc:else> Statement 3 </template> </template>
4. Dynamically Pass Bind Variables to a SOQL Query: With the new Database.queryWithBinds
, Database.getQueryLocatorWithBinds
, and Database.countQueryWithBinds
methods, the bind variables in the query are resolved from a Map parameter directly with a key rather than from Apex code variables. As a result, it’s not necessary for the variables to be in scope when the query is executed.
Map<String, Object> acctBinds = new Map<String, Object>{'acctName' => 'Acme Corporation'}; List<Account> accts = Database.queryWithBinds('SELECT Id FROM Account WHERE Name = :acctName', acctBinds, AccessLevel.USER_MODE);
5. Specify an Org-Wide Default Delay in Scheduling Queueable Jobs: Admins can define a default delay (1–600 seconds) in scheduling queueable jobs that were scheduled without a delay parameter. Use the delay setting as a mechanism to slow default queueable job execution. If the setting is omitted, Apex uses the standard queueable timing with no added delay.
From Setup, in the Quick Find box, enter Apex Settings, and then enter a value (1–600 seconds) for the Default minimum enqueue delay (in seconds) for queueable jobs that do not have a delay parameter.
6. Use the System.enqueueJob Method to Specify a Delay in Scheduling Queueable Jobs: A new optional override adds queueable jobs to the asynchronous execution queue with a specified minimum delay (0–10 minutes). Using the System.enqueue(queueable, delay)
method ignores any org-wide enqueue delay setting. The delay is ignored during Apex testing.
Integer delayInMinutes = 10; ID jobID = System.enqueueJob(new MyQueueableClass(), delayInMinutes);
7. Restrict Anonymous Apex Access to Core Users: By using the RestrictCommunityExecAnon preference, admins can restrict anonymous Apex execution to core users, such as Standard and CsnOnly users. When enabled, noncore users can’t execute anonymous Apex regardless of any other user permissions that are set, such as Author Apex.
From Setup, in the Quick Find box, enter Apex Settings, and then select Restrict non-core users from executing anonymous Apex.
8. Secure Apex Code with User Mode Database Operations : he new Database
and Search
methods support an accessLevel
parameter that lets you run database and search operations in user mode instead of in the default system mode. To enhance the security context of Apex, you can specify user mode access for database operations. Field-level security (FLS) and object permissions of the running user are respected in user mode and it always applies sharing rules.
//when we query the data List<Account> acc = [SELECT Id FROM Account WITH USER_MODE]; //For DML Operation Account acc = new Account(Name='test'); insert as user acc;
9. Get Accessibility Changes to Lightning Design System Icons: There’s a slight change in color to Salesforce Lightning Design System (SLDS) icons. If you use static SLDS Standard Object, Action, or Doctype icons, you must update your files.
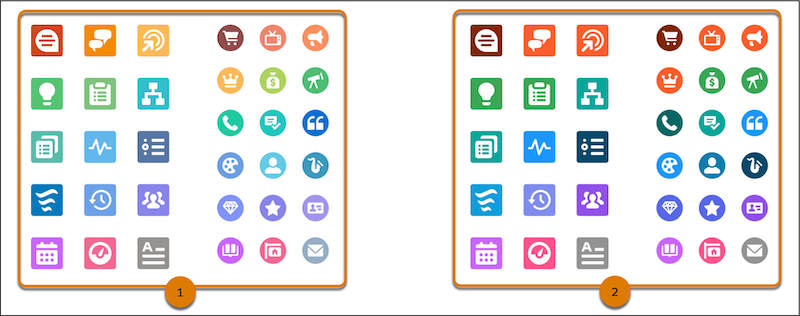
10. Fetch Data Using the GraphQL Wire Adapter (Pilot): Built on the Salesforce GraphQL API, the GraphQL wire adapter enables you to use UI API-enabled objects with the object-level security and field-level security of the current user. The wire adapter is quipped with client-side caching and data management capabilities provided by Lightning Data Service.
import { LightningElement, wire } from 'lwc'; import { gql, graphql } from 'lightning/uiGraphQLApi'; export default class ExampleGQL extends LightningElement { @wire(graphql, { query: gql` query AccountInfo { uiapi { query { Account(where: { Name: { like: "United%" } }) @category(name: "recordQuery") { edges { node { Name @category(name: "StringValue") { value displayValue } } } } } } }` }) propertyOrFunction }
11. Track Field History for Activities like Events and Tasks : Now we can track events and task field changes history. Track up to six fields so that sales reps can see what’s changed in the Related tab for events and tasks.
12. Convert Processes to Flows with the Migrate to Flow Tool: The updated Migrate to Flow tool will support the migration of Process Builder. Previously only available to migrate Workflow Rules, you can now use the tool to convert Process Builder processes to Flows.
13. Synchronize Component Data Without a Page Refresh Using RefreshView API (Beta): Whether user-driven or app-invoked, the ability to synchronize data without reloading an entire page is a key user experience requirement. The new lightning/refresh
module and RefreshView API provide a standard way to refresh component data in LWC and Aura. Previously, LWC lacked a data refresh API, and Aura only supported the legacy force:refreshView
, which doesn’t meet the requirements of modern web development. RefreshView API’s detailed control of refresh scope lets developers create refined user experiences while maintaining backward compatibility.
14. Add Lookup Fields to Your Flow Screens with Ease: Easily add Lookup fields to flow screens and create a record directly from the Lookup field with Dynamic Forms for Flow. On the Records tab, hover over a Lookup field from your record resource, and then drag in your desired Lookup field.
15. See Element Descriptions on the Flow Canvas: Now you can easily see what each element is doing within a flow in Auto-Layout. Previously, to see the user-provided description, you opened the element.
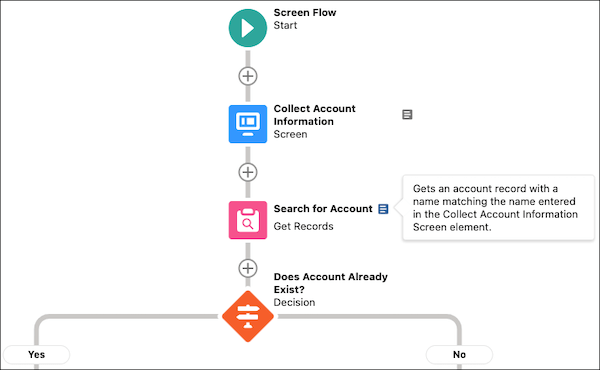