Hello friends, today I am going to discuss Set default fields values on create record LWC Salesforce. We can create records with default values while using standard record-form navigation.
Also, check this: Generate QR codes for Salesforce Object
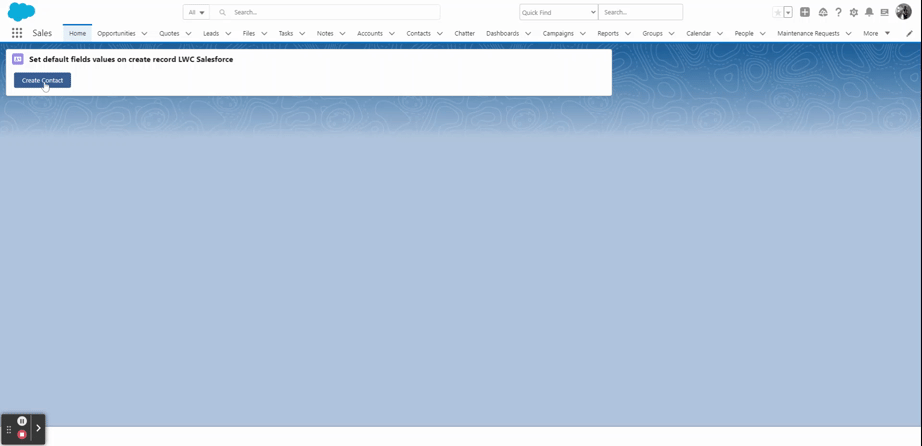
Key Highlights :
- Setup default field values before opening the record form.
Code :
For the demo purpose, I have created a basic component that will populate the default values in the Contact record.
Lwcform.Html:
<template>
<lightning-card title="Set default fields values on create record LWC Salesforce" icon-name="standard:contact">
<div class="slds-m-around_medium">
<lightning-button label="Create Contact" variant="brand" onclick={createRecordWithDefaultValues}></lightning-button>
</div>
</lightning-card>
</template>
Lwcform.JS:
import { LightningElement } from 'lwc';
import { NavigationMixin } from 'lightning/navigation';
import { encodeDefaultFieldValues } from 'lightning/pageReferenceUtils';
export default class Lwcform extends NavigationMixin(LightningElement) {
createRecordWithDefaultValues() {
//Object will be having field names and values
const defaultValues = encodeDefaultFieldValues({
FirstName: 'TechDicer',
LastName: 'KeepLearning',
LeadSource: 'Web',
Email:'test@g.com',
Title:'Techdicer'
});
this[NavigationMixin.Navigate]({
type: 'standard__objectPage',
attributes: {
objectApiName: 'Contact',
actionName: 'new'
},
state: {
defaultFieldValues: defaultValues
}
});
}
}
Output :
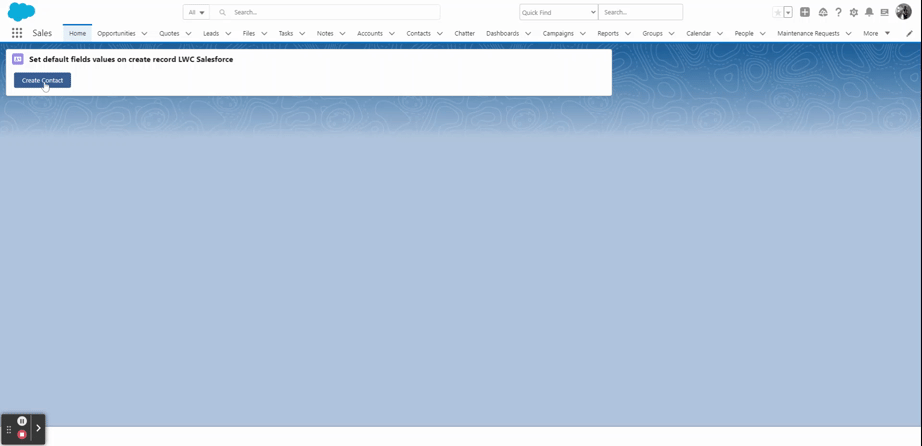
Reference :
What’s your Reaction?
+1
1
+1
+1
1
+1
+1
+1
7 comments
Hi, this is Amazing Post
thank you @MOHAMMAD AHTESHAM
Hi and thanks for your nice post. I wonder how to set a default value for a field with picklist data type based on a criteria? I mean conditional default value. For instance, we want that a picklist value default be XXX if field1 is YYY AND field2 is zzz.
Hi Mary, you can create a variable and put your conditions so you can get a variable value and then set it in encodeDefaultFieldValues({
in createRecordWithDefaultValues method
Like :
createRecordWithDefaultValues() {
let firstname = ”;
if(compName == ‘TechDicer’){
firstname = ‘TechDicer’;
}
//Object will be having field names and values
const defaultValues = encodeDefaultFieldValues({
FirstName: firstname,
LastName: ‘KeepLearning’,
LeadSource: ‘Web’,
Email:’test@g.com’,
Title:’Techdicer’
});
Thanks Rijwan! I’ll try it.
Hi, Can we override, this record form’s Save button?
We can handle this save button click,
HTML
JS
onSubmitHandler(event) {
event.preventDefault();
// Get data from submitted form
const fields = event.detail.fields;
// Here you can execute any logic before submit
// and set or modify existing fields
fields.Name = fields.Name + fields.Phone
// You need to submit the form after modifications
this.template
.querySelector('lightning-record-edit-form').submit(fields);
}